Word2Vec#
by Erfan Fakoor
Contact : e.f.simorgh@gmail.com
Pattern Recognition - Octobor 2024
In this reading, we want to get acquainted with the concept of Word2Vec. let’s start with a quote:
“There is in all things a pattern that is part of our universe. It has symmetry, elegance, and grace - those qualities you find always in that which the true artist captures. You can find it in the turning of the seasons, in the way sand trails along a ridge, in the branch clusters of the creosote bush or the pattern of its leaves. We try to copy these patterns in our lives and our society, seeking the rhythms, the dances, the forms that comfort. Yet, it is possible to see peril in the finding of ultimate perfection. It is clear that the ultimate pattern contains it own fixity. In such perfection, all things move toward death” ~Dune by Frank Herbert (1965)
Learning Goals#
Introduction to Word Embeddings
Introduction to Language Modeling
Familiarization with Language Model Training
Introduction to Word2Vec
Understanding How Word2Vec is Trained
Familiarization with Different Structures of Word2Vec
Familiarization with the Word2Vec Training Process
Working with Word2Vec in Python
Understanding Why Word2Vec is Revolutionary
Understanding the Limitations of Word2Vec
Understanding the Applications of W2Vec
Introduction#
The concept of embeddings is one of the most intriguing aspects of machine learning, especially in the context of Natural Language Processing (NLP). When we use Siri, Google Assistant, Alexa, Google Translate, or a smartphone keyboard with predictive text, we likely experience the power of embeddings. Over the past couple of decades, there has been significant progress in applying embeddings to neural models, with recent advancements such as contextualized word embeddings driving state-of-the-art models like BERT and GPT-2.
Word2vec, introduced in 2013, is a highly efficient method for creating word embeddings. Beyond its primary use in Natural Language Processing (NLP), some of its underlying concepts have proven effective for building recommendation engines and analyzing sequential data in non-language-related tasks. Major companies such as Airbnb, Alibaba, Spotify, and Anghami have leveraged this powerful tool, applying it in production to create a new generation of recommendation systems.
Now, we’ll explore the idea of embeddings and the process of generating them with word2vec. But first, let’s start with a simple example to illustrate how vectors can represent various things.
Personality Embeddings: What are you like?#
Did you know that a set of five numbers (a vector) can reveal a lot about your personality? On a scale from 0 to 100, where 0 represents extreme introversion and 100 represents extreme extraversion, how would you rate yourself? Have you ever taken a personality test like the MBTI or, even better, the Big Five Personality Traits test? If not, these tests ask a series of questions and evaluate you across different dimensions, with introversion/extraversion being one of them.
Suppose Jay scored 38/100 as his introversion/extraversion score. we can plot that in this way:
Let’s change the range to be from -1 to 1:
How well do we feel we know Jay knowing only this one piece of information about him? Not much. People are complex. So let’s add another dimension – the score of one other trait from the test.
We can represent the two dimensions as a point on the graph, or better yet, as a vector from the origin to that point.
In this plots we purposely hidden which traits are being plotted so you can get accustomed to interpreting the vector representation of a person’s personality without knowing exactly what each dimension stands for – yet still gaining valuable insights. At this point, we can say that this vector captures a part of Jay’s personality. The strength of such a representation becomes evident when you want to compare others to Jay. For instance, if Jay were suddenly hit by a bus and needed to be replaced by someone with a similar personality, which of the two individuals shown in the figure below would be a closer match to him?
When dealing with vectors, a common way to calculate a similarity score is cosine similarity. Maybe you ask “What is cosine similarity?” In data analysis, cosine similarity is a measure of similarity between two non-zero vectors defined in an inner product space. Cosine similarity is the cosine of the angle between the vectors; that is, it is the dot product of the vectors divided by the product of their lengths. Read More.
Person #1 is more similar to Jay in personality. Vectors pointing at the same direction (length plays a role as well) have a higher cosine similarity score.
Once again, two dimensions aren’t sufficient to fully capture the complexity of how different people are. After decades of psychological research, five major personality traits (with many sub-traits) have been identified. So, let’s incorporate all five dimensions into our comparison.
The challenge with using five dimensions is that we can no longer easily visualize the data with simple arrows in two dimensions. This is a common issue in machine learning, where we often need to think in higher-dimensional spaces. Fortunately, cosine similarity still functions well, regardless of the number of dimensions.
By the end of this intro, we grasped two key ideas:
We can represent people (and objects) as vectors of numbers (which is very useful for machines).
We can easily compute how similar these vectors are to one another.
Word Embeddings#
With this foundation, we can now explore examples of trained word vectors, also known as word embeddings, and examine some of their fascinating characteristics. Here’s an example of a word embedding for the word “king” (a GloVe vector trained on Wikipedia):
The list consists of 50 numbers, but just by looking at them, it’s hard to draw any meaningful conclusions. To make comparisons with other word vectors easier, let’s visualize the data. We’ll arrange all the numbers in a single row and use color coding: red for values near 2, white for values close to 0, and blue for values around -2.
We’ll proceed by ignoring the numbers and only looking at the colors to indicate the values of the cells. Let’s now contrast “King” against other words. See how “Man” and “Woman” are much more similar to each other than either of them is to “king”? This tells you something. These vector representations capture quite a bit of the information/meaning/associations of these words.
Here are a few observations:
There’s a consistent red column across all the different words, indicating they are similar along that dimension, though we don’t know what each dimension represents.
“Woman” and “girl” share similarities in several areas, as do “man” and “boy.”
“Boy” and “girl” also have commonalities that set them apart from “woman” and “man.” Could this dimension represent a general concept of youth? It’s possible.
All the words, except the last one, represent people. I included an object (“water”) to highlight differences between categories. For instance, you can see a blue column that extends down and stops before the “water” embedding.
There are clear areas where “king” and “queen” are similar to each other but distinct from the other words. Could this be encoding a concept of royalty?
Analogies#
One of the most remarkable properties of embeddings is their ability to handle analogies. By adding and subtracting word embeddings, we can obtain intriguing results. A well-known example is the formula: “king” - “man” + “woman.” Using the Gensim library in Python, we can perform these operations on word vectors and identify the words most similar to the resulting vector. The image displays a list of these similar words, along with their cosine similarity scores.
We can visualize this analogy as we did previously:
The vector produced by “king - man + woman” isn’t an exact match for “queen,” but among the 400,000 word embeddings in this collection, “queen” is the word most similar to it.
Having explored trained word embeddings, let’s dive into the training process. Before we examine word2vec, though, we first need to understand a key precursor to word embeddings: the neural language model.
Language Modeling#
A great example of an NLP application is the next-word prediction feature found in smartphone keyboards—a tool billions of people use hundreds of times daily.
Next-word prediction is tackled by a language model, which can take a sequence of words (for instance, two words) and try to predict the next word.
In the screenshot above, we can think of the model as one that took in these two green words (thou shalt) and returned a list of suggestions (“not” being the one with the highest probability):
We can visualize the model as a “black box”:
However, in reality, the model doesn’t predict just one word. Instead, it generates a probability score for every word in its vocabulary, which can range from a few thousand to over a million words. The keyboard application then selects the words with the highest probability and suggests them to the user.
The output of the neural language model is a probability score for all the words the model knows. We’re referring to the probability as a percentage here, but 40% would actually be represented as 0.4 in the output vector.
Early neural language models (Bengio, 2003) made predictions through a three-step process after being trained.
The first step, which is most relevant when discussing embeddings, produced a matrix with an embedding for each word in the vocabulary. During prediction, we simply retrieve the embeddings for the input words and use them to make predictions:
Now, let’s dive into the training process to better understand how this embedding matrix is created.
Language Model Training#
One major advantage language models have over many other machine learning models is that they can be trained on vast amounts of existing text—like books, articles, and Wikipedia entries—without needing specially curated data or handcrafted features.
“You shall know a word by the company it keeps” J.R. Firth
Word embeddings are learned by observing which other words commonly appear nearby. The process works as follows:
We gather a large text corpus (e.g., all Wikipedia articles).
We apply a sliding window (say, three words at a time) across the text.
This sliding window generates training samples for the model.
As the window moves through the text, it (virtually) produces a dataset for training. To illustrate, let’s look at how the sliding window processes a phrase. Initially, the window captures the first three words of the sentence:
The first two words act as features, while the third is the label:
The window then moves to the next set of words, creating a new training sample:
Before long, we’ve generated a large dataset showing which words tend to follow certain pairs of words:
In practice, models are typically trained as the window slides through the text, but I find it helpful to conceptually separate the “dataset generation” phase from the training phase. Prior to neural-network-based language models, N-grams were a commonly used technique. For a real-world example of this transition from N-grams to neural models, check out a 2015 blog post from Swiftkey, which introduced their neural language model and compared it with their previous N-gram model. This example is interesting because it demonstrates how the technical properties of embeddings can be explained in marketing terms.
Knowing what you know from earlier in the post, fill in the blank:
In the example I provided, you had five words before the blank (and a previous mention of “bus”). Most people would likely guess that “bus” fits in the blank. But what if I gave you another word after the blank—would that change your answer?
This additional information completely alters what should fill the blank. Now, “red” becomes the most likely choice. What this teaches us is that both the words before and after a specific word hold valuable information. By considering both directions—words to the left and right of the target word—we can create more accurate word embeddings. Let’s explore how we can modify the training process to incorporate this.
How is Word2Vec trained?#
Word2Vec is trained using a neural network that learns word relationships from large text datasets. To represent a word as a vector in multidimensional space, the algorithm operates in one of two structures: Continuous Bag of Words (CBOW) or Skipgram.
Continuous Bag of Words (CBOW)#
Instead of only looking at the two words before the target word, we can also consider the two words following it.
By doing this, the dataset we are virtually building to train the model would look like this:
This approach is known as the Continuous Bag of Words (CBOW) architecture.
Let’s say we want each word in a corpus to be predicted by every other word within a small span of four words. We define the neighboring word set as \(N={-4,-3,-2,-1,+1,+2,+3,+4}\). The training objective is to maximize the following expression:
In other words, we aim to maximize the overall probability for the entire corpus using a probability model that predicts words based on their neighbors.
To avoid the numerical instability of products, we take the logarithm, turning the objective into maximizing the log-probability of the corpus.
That is, we maximize the log-probability of the corpus. The probability model works as follows: Given neighboring words \({w_j : j \in N+i}\), it calculates their vector sum \(v:=\sum_{j \in N+i}v_{w_j}\), then performs a dot-product-softmax with other vectors (similar to the attention mechanism in Transformers) to obtain the probability:
The goal is to maximize this quantity:
While the left side is quick to compute, the right side is much slower, as it requires summing over the entire vocabulary for each word in the corpus. Additionally, using gradient ascent to maximize the log-probability involves computing the gradient of the right-hand quantity, which is computationally intractable. To address this, the authors implemented numerical approximation techniques.
Skipgram#
Another architecture that has also demonstrated strong results approaches the task a bit differently. Instead of predicting a word based on its context (the words before and after it), this architecture attempts to predict the neighboring words using the current word.
We can visualize the sliding window for this method like this:
The word in the green slot would be the input word, each pink box would be a possible output.
The pink boxes vary in shade because the sliding window generates four separate training samples:
This approach is known as the skipgram architecture. We can imagine the sliding window functioning as follows:
These four samples are added to our training dataset:
Next, we slide the window to the following position:
Which produces four more examples:
After a few more positions, we’ve accumulated many examples:
For skip-gram, the training objective is to maximize the total probability for the corpus by predicting each word’s neighbors independently. $\(\prod_{i \in C}Pr(w_i : j \in N+i | w_i)\)\( \)\(Pr(w_j:j \in N+i | w_i) = \prod_{j \in N+i}Pr(w_j | w_i)\)$ To avoid the numerical instability of products, we take the logarithm, turning the objective into maximizing the log-probability of the corpus.
The probability model still uses the dot-product-softmax, so the calculation process remains the same.
The main difference between skip-gram and CBOW lies in this equation.
Now we probably understand that the skipgram model is the inverse of the CBOW model. Instead of using surrounding words to predict the center word, it uses the center word to predict the surrounding context. The choice between these two approaches depends on the task. Skip-gram excels with smaller datasets and performs well with infrequent words, while CBOW is more effective for frequently occurring words.
Revisiting the training process#
Now that we have our skipgram training dataset from the existing text, let’s look at how it’s used to train a simple neural language model to predict neighboring words.
We begin with the first sample in our dataset, taking the input (feature) and feeding it to the untrained model, asking it to predict a neighboring word.
The model goes through three steps and produces a prediction vector, assigning a probability to each word in its vocabulary. Since it’s untrained, the prediction will likely be incorrect, but that’s expected. We already know the correct word (the label in the current row of our training data):
The ‘target vector’ is one where the target word has the probability 1, and all other words have the probability 0.
To measure the model’s error, we subtract the predicted vector from the actual target vector, resulting in an error vector:
This error vector helps adjust the model so that next time, it’s a bit more likely to predict the correct word (e.g., predicting “thou” when given “not” as input).
That completes the first step of training. We repeat this process for the next sample in the dataset, and the next, until we’ve processed all the samples. This completes one training epoch. We continue for several epochs until the model is trained, at which point we can extract the embedding matrix for other applications.
While this explains the basic process, there are still a few key concepts missing from the way word2vec is actually trained.
Negative Sampling#
Remember the three steps in how the neural language model makes predictions.
The third step is particularly computationally expensive, especially when we have to repeat it for every training sample in our dataset, which can be millions of times. To improve efficiency, we can break down the task into two steps:
Generate high-quality word embeddings (without focusing on next-word prediction).
Use these embeddings to train a language model for next-word prediction.
In this explanation, we’ll focus on the first step—creating effective embeddings. To do this, instead of predicting a neighboring word, we change the task. The model will now take an input and an output word and simply output a score to determine whether they are neighbors (1 for neighbors, 0 for non-neighbors).
This adjustment simplifies the task, allowing us to replace the neural network with a logistic regression model, which is much faster and simpler to compute.
However, we also need to restructure our dataset to reflect this change. The labels will now be a new column indicating whether the words are neighbors (1) or not (0). Initially, all examples will be positive (1), since the pairs we add are neighboring words.
To avoid creating a model that always predicts 1, we need to add negative samples—pairs of words that aren’t neighbors, where the label should be 0. This forces the model to distinguish between true neighbors and randomly paired words, making it a more challenging (and useful) task.
For each sample in our dataset, we add negative examples. Those have the same input word, and a 0 label.
The negative samples are generated by randomly selecting words from our vocabulary, and this technique is inspired by Noise-Contrastive Estimation (Read more about this), which balances computational speed and statistical accuracy by contrasting actual neighbors with random noise.
Skipgram with Negative Sampling (SGNS)#
We’ve now introduced two key concepts in word2vec: skip-gram and negative sampling. Together, they form the skip-gram with negative sampling model. With these foundational ideas in place, we can delve into the word2vec training process.
Word2vec Training Process#
Before training begins, we preprocess the text to establish the vocabulary size (let’s call it vocab_size, for instance, 10,000) and identify the words in the vocabulary.
Once training starts, two matrices are created: the Embedding matrix and the Context matrix. Each of these matrices contains an embedding for every word in the vocabulary. The size of the embeddings (referred to as embedding_size) is the second dimension, with common sizes being 300 or 50, as discussed earlier.
At the start, both matrices are initialized with random values. During each training step, we take a positive example (an input word and its neighboring word) along with negative samples (words that are not neighbors).
For example, the input word might be “not,” with “thou” as its actual neighbor, and “aaron” and “taco” as negative samples. We then look up the embeddings of the input word from the Embedding matrix and the context words from the Context matrix.
Next, we compute the dot product between the input word embedding and each context word embedding, producing a similarity score for each pair.
These scores are then passed through the sigmoid function to transform them into probabilities, which are values between zero and one.
Now, using the actual labels (1 for neighbors, 0 for non-neighbors), we compare these probabilities with the true labels by calculating the error (the difference between the predicted probabilities and the target values).
This error allows the model to learn. We use it to update the embeddings of the input and context words (“not,” “thou,” “aaron,” and “taco”), so that next time, the model’s predictions will be closer to the target values.
This process repeats for the next positive sample and its corresponding negative samples, and it continues as we cycle through the entire dataset multiple times.
Eventually, we stop the training, discard the Context matrix, and retain the Embedding matrix as our final pre-trained word embeddings, ready for use in other tasks.
Window Size and Number of Negative Samples#
Two important hyperparameters in the word2vec training process are the window size and the number of negative samples.
The window size affects how the model learns word relationships. Smaller window sizes (2-15) tend to produce embeddings where high similarity between two words suggests they can be used interchangeably. For example, antonyms like “good” and “bad” may often appear in similar contexts and thus have similar embeddings. On the other hand, larger window sizes (15-50 or more) result in embeddings that reflect a broader sense of relatedness between words rather than interchangeability. In practice, annotations can guide the embeddings to create meaningful similarity for your specific task. By default, Gensim uses a window size of 5, meaning it looks at five words before and after the target word, plus the word itself.
Another key factor is the number of negative samples. According to the original word2vec paper, 5-20 negative samples work well, though 2-5 may suffice if the dataset is large enough. Gensim’s default setting is 5 negative samples.
Word2Vec in Python#
We can generate word embeddings for our corpus in Python using the genism module. First step is Installing modules, We start by installing the ‘gensim’ and ‘nltk’ modules.
pip install gensim
pip install nltk
We can effortlessly train word2vec embeddings using Gensim, an open-source Python library designed to represent documents as semantic vectors in a way that is both computationally efficient and user-friendly.
For this demonstration, I used the Coronavirus tweets NLP dataset from Kaggle. I chose this over larger datasets, such as IMDB, because many of our applications work with datasets of similar size, making it a better example of the model’s typical performance.
import numpy as np
import matplotlib.pyplot as plt
import pandas as pd
dataset = pd.read_csv('./assets/Corona_NLP_train.csv', encoding='latin1')
import re
texts=[]
for i in range(0,len(dataset)):
text = re.sub('[^a-zA-Z]' , ' ', dataset['OriginalTweet'][i])
text = text.lower()
text = text.split()
x = len(text) if text.count('https') ==0 else text.index('https')
text = text[: x ]
text = [t for t in text if not t=='https']
text = ' '.join(text)
texts.append(text)
from gensim.models import Word2Vec
sentences = [line.split() for line in texts]
w2v =Word2Vec(sentences, vector_size=100, window=5, workers=4, epochs=10, min_count=5)
print(sentences[20:25]) #Training the word2vec model
---------------------------------------------------------------------------
ModuleNotFoundError Traceback (most recent call last)
Cell In[1], line 2
1 import numpy as np
----> 2 import matplotlib.pyplot as plt
3 import pandas as pd
5 dataset = pd.read_csv('./assets/Corona_NLP_train.csv', encoding='latin1')
ModuleNotFoundError: No module named 'matplotlib'
Explanation#
Finding the vocabulary of the model provides us with a list of words we can try and use other functions:
words = list(w2v.wv.index_to_key)
print(words)
['the', 'to', 'and', 'of', 'covid', 'a', 'in', 'coronavirus', 'for', 'is', 'are', 'i', 'you', 'on', 's', 'this', 'prices', 'at', 'it', 'food', 'supermarket', 'we', 'store', 'that', 'with', 'grocery', 'have', 'as', 'be', 'people', 't', 'from', 'amp', 'all', 'can', 'consumer', 'your', 'not', 'will', 'they', 'my', 'our', 'up', 'out', 'has', 'or', 'by', 'more', 'but', 'shopping', 'if', 'online', 'how', 'their', 'during', 'pandemic', 'so', 'now', 'no', 'what', 'get', 'about', 'who', 'need', 'workers', 'panic', 'just', 'there', 'us', 'do', 'like', 'time', 'sanitizer', 'home', 'was', 'an', 'demand', 'go', 'when', 'some', 'help', 'hand', 'stock', 'don', 're', 'going', 'one', 'm', 'me', 'here', 'due', 'buying', 'been', 'oil', 'new', 'crisis', 'please', 'work', 'them', 'after', 'other', 'toilet', 'because', 'only', 'these', 'should', 'toiletpaper', 'than', 'paper', 'today', 'over', 'stay', 'local', 'retail', 'keep', 'buy', 'stores', 'many', 'shelves', 'still', 'being', 'make', 'delivery', 'those', 'down', 'see', 'day', 'know', 'why', 'take', 'lockdown', 'supply', 'outbreak', 'would', 'stop', 'its', 'uk', 'staff', 'into', 've', 'virus', 'social', 'everyone', 'could', 'any', 'had', 'even', 'masks', 'price', 'while', 'health', 'working', 'essential', 'also', 'week', 'world', 'market', 'think', 'good', 'business', 'amid', 'spread', 'he', 'use', 'shop', 'customers', 'were', 'safe', 'most', 'way', 'thank', 'am', 'government', 'off', 'employees', 'which', 'socialdistancing', 'right', 'impact', 'every', 'products', 'back', 'much', 'before', 'news', 'first', 'may', 'items', 'doing', 'supplies', 'times', 'last', 'mask', 'free', 'where', 'support', 'read', 'well', 'days', 'face', 'high', 'low', 'want', 'around', 'find', 'got', 'coronacrisis', 'then', 'global', 'distancing', 'quarantine', 'through', 'said', 'let', 'weeks', 'supermarkets', 'u', 'gas', 'public', 'risk', 'corona', 'empty', 'too', 'open', 'says', 'country', 'getting', 'really', 'money', 'went', 'very', 'economy', 'since', 'companies', 'she', 'businesses', 'consumers', 'long', 'care', 'order', 'say', 'two', 'making', 'things', 'next', 'her', 'line', 'enough', 'great', 'his', 'look', 'others', 'full', 'll', 'available', 'march', 'hands', 'service', 'check', 'pay', 'family', 'via', 'services', 'hours', 'shops', 'gloves', 'taking', 'chain', 'against', 'anyone', 'medical', 'come', 'put', 'response', 'across', 'year', 'must', 'industry', 'china', 'trump', 'd', 'house', 'life', 'increase', 'few', 'state', 'needs', 'everything', 'hoarding', 'goods', 'fight', 'markets', 'closed', 'self', 'protect', 'big', 'trying', 'without', 'same', 'etc', 'best', 'report', 'groceries', 'emergency', 'never', 'increased', 'already', 'economic', 'community', 'amazon', 'thing', 'vulnerable', 'sure', 'close', 'elderly', 'per', 'drivers', 'live', 'give', 'post', 'thanks', 'small', 'made', 'place', 'did', 'each', 'someone', 'real', 'scams', 'india', 'call', 'sales', 'seen', 'having', 'better', 'such', 'situation', 'continue', 'shoppers', 'banks', 'latest', 'using', 'behavior', 'does', 'lot', 'job', 'another', 'hard', 'person', 'looking', 'cases', 'hope', 'away', 'bank', 'morning', 'healthcare', 'part', 'share', 'important', 'hit', 'avoid', 'water', 'together', 'coming', 'selling', 'gt', 'left', 'under', 'data', 'able', 'own', 'n', 'production', 'front', 'retailers', 'coronaviruspandemic', 'daily', 'drop', 'tesco', 'nhs', 'protection', 'learn', 'stayhome', 'change', 'run', 'information', 'normal', 'company', 'spending', 'end', 'ever', 'current', 'list', 'essentials', 'until', 'key', 'less', 'measures', 'won', 'else', 'positive', 'again', 'might', 'start', 'least', 'coronavirusoutbreak', 'pm', 'outside', 'feel', 'safety', 'financial', 'related', 'milk', 'man', 'wash', 'remember', 'leave', 'done', 'police', 'told', 'jobs', 'seeing', 'little', 'thought', 'nothing', 'years', 'tips', 'w', 'months', 'stayathome', 'shortage', 'cut', 'wearing', 'lives', 'stayhomesavelives', 'something', 'old', 'month', 'meet', 'yet', 'fall', 'farmers', 'restaurants', 'keeping', 'families', 'contact', 'helping', 'sick', 'video', 'worker', 'including', 'april', 'doesn', 'e', 'access', 'reports', 'soap', 'update', 'doctors', 'roll', 'isolation', 'travel', 'wear', 'chains', 'panicbuying', 'isn', 'running', 'stophoarding', 'rise', 'alcohol', 'distance', 'lower', 'americans', 'possible', 'try', 'higher', 'bad', 'used', 'always', 'city', 'needed', 'instead', 'non', 'lines', 'b', 'media', 'nurses', 'found', 'set', 'yourself', 'based', 'number', 'soon', 'likely', 'provide', 'major', 'visit', 'stocked', 'hospital', 'prevent', 'bought', 'changes', 'pharmacy', 'shortages', 'once', 'follow', 'offering', 'watch', 'drive', 'didn', 'love', 'anything', 'advice', 'relief', 'tp', 'k', 'testing', 'putting', 'govt', 'sanitizers', 'hour', 'produce', 'gouging', 'between', 'yesterday', 'selfish', 'friends', 'far', 'walmart', 'especially', 'orders', 'coronavirusupdate', 'advantage', 'affected', 'almost', 'aren', 'gone', 'deal', 'healthy', 'states', 'ensure', 'special', 'car', 'million', 'brands', 'whole', 'paid', 'meat', 'understand', 'join', 'th', 'saw', 'customer', 'top', 'sell', 'result', 'reduce', 'following', 'russia', 'system', 'eat', 'staying', 'kids', 'fear', 'control', 'realdonaldtrump', 'area', 'show', 'credit', 'test', 'means', 'website', 'fresh', 'actually', 'tested', 'war', 'stoppanicbuying', 'remain', 'article', 'countries', 'bread', 'save', 'heroes', 'surge', 'shut', 'below', 'story', 'y', 'tell', 'case', 'die', 'info', 'despite', 'wait', 'canada', 'works', 'sold', 'rolls', 'caused', 'stuff', 'point', 'cannot', 'ask', 'huge', 'announced', 'australia', 'extra', 'america', 'woman', 'cost', 'kind', 'cash', 'saying', 'waiting', 'critical', 'trip', 'themselves', 'maybe', 'moment', 'three', 'period', 'early', 'given', 'italy', 'asked', 'yes', 'limit', 'changing', 'security', 'difficult', 'restaurant', 'c', 'ago', 'facing', 'idea', 'clean', 'shit', 'product', 'struggling', 'queue', 'employee', 'eggs', 'drug', 'become', 'cleaning', 'personal', 'short', 'staysafe', 'fuel', 'both', 'taken', 'him', 'basic', 'increasing', 'mean', 'national', 'pick', 'bring', 'ways', 'spreading', 'scammers', 'lost', 'seriously', 'nation', 'fast', 'tomorrow', 'different', 'crazy', 'currently', 'sector', 'shift', 'inside', 'consider', 'president', 'purchase', 'experts', 'survive', 'term', 'started', 'trends', 'changed', 'sainsburys', 'restrictions', 'research', 'quarantinelife', 'energy', 'cause', 'stockpiling', 'future', 'ppl', 'giving', 'closing', 'buyers', 'gold', 'deliveries', 'guys', 'offer', 'act', 'further', 'reduced', 'allowed', 'group', 'issues', 'stocks', 'large', 'sign', 'action', 'shows', 'behaviour', 'asda', 'team', 'american', 'makes', 'hey', 'recent', 'donate', 'fears', 'office', 'plan', 'providing', 'school', 'rs', 'r', 'ppe', 'trade', 'wrong', 'confidence', 'asking', 'recession', 'london', 'fucking', 'alert', 'starting', 'closures', 'concerns', 'plenty', 'folks', 'marketing', 'law', 'usa', 'strong', 'st', 'walk', 'problem', 'general', 'wipes', 'direct', 'crude', 'near', 'goes', 'pasta', 'thinking', 'falling', 'gonna', 'ready', 'continues', 'worried', 'ones', 'worse', 'household', 'past', 'came', 'living', 'link', 'gov', 'bit', 'according', 'limited', 'raise', 'chinese', 'longer', 'door', 'afford', 'hear', 'though', 'deliver', 'questions', 'probably', 'fighting', 'god', 'behind', 'expected', 'believe', 'half', 'haven', 'saudi', 'ceo', 'resources', 'single', 'borisjohnson', 'night', 'expect', 'fuck', 'equipment', 'email', 'died', 'citizens', 'federal', 'allow', 'happy', 'necessary', 'monday', 'send', 'poor', 'looks', 'delivered', 'friend', 'members', 'hospitals', 'bottle', 'updates', 'income', 'insights', 'side', 'experience', 'rules', 'parents', 'ok', 'ecommerce', 'truck', 'frontline', 'p', 'nice', 'county', 'efforts', 'seems', 'message', 'rice', 'lots', 'anxiety', 'forced', 'foods', 'pack', 'places', 'sale', 'tests', 'light', 'co', 'coronavirusuk', 'hoard', 'symptoms', 'called', 'plans', 'society', 'easter', 'reason', 'rising', 'question', 'unprecedented', 'effects', 'potential', 'digital', 'issue', 'seniors', 'blog', 'gets', 'kill', 'survey', 'human', 'serious', 'apart', 'comes', 'calls', 'habits', 'slots', 'ebay', 'took', 'south', 'however', 'amount', 'biggest', 'listen', 'opening', 'responders', 'finally', 'phone', 'inflated', 'california', 'interesting', 'usual', 'street', 'infected', 'nurse', 'bags', 'hygiene', 'death', 'effect', 'click', 'move', 'hiking', 'worth', 'massive', 'wake', 'beginning', 'residents', 'morrisons', 'talk', 'collapse', 'driving', 'level', 'handsanitizer', 'rather', 'several', 'temporarily', 'coughing', 'disease', 'clear', 'policy', 'growing', 'washing', 'distribution', 'sentiment', 'donations', 'dear', 'true', 'shelf', 'cure', 'dont', 'bill', 'debt', 'homes', 'record', 'rent', 'forget', 'communities', 'pretty', 'cheap', 'happen', 'amazing', 'oh', 'touch', 'x', 'ftc', 'force', 'children', 'breaking', 'hoarders', 'couple', 'rate', 'details', 'hold', 'opec', 'among', 'myself', 'profit', 'unemployment', 'hi', 'step', 'growth', 'calling', 'feed', 'insurance', 'spend', 'showing', 'guess', 'dr', 'fake', 'easy', 'shame', 'rt', 'toiletpapercrisis', 'minister', 'vegetables', 'profiteering', 'tonight', 'commerce', 'impacted', 'costs', 'f', 'giant', 'regarding', 'later', 'o', 'stand', 'donating', 'second', 'calm', 'power', 'quick', 'challenges', 'sir', 'simple', 'commodities', 'turn', 'g', 'fruit', 'package', 'mind', 'department', 'priority', 'meals', 'talking', 'deaths', 'card', 'dropped', 'wine', 'four', 'benefits', 'worry', 'notice', 'note', 'thousands', 'worst', 'bulk', 'ahead', 'paying', 'webinar', 'impacts', 'united', 'quickly', 'clerks', 'eating', 'head', 'weekly', 'imagine', 'takes', 'plus', 'along', 'protective', 'stimulus', 'mass', 'producers', 'age', 'scam', 'housing', 'certain', 'date', 'rest', 'groups', 'aisles', 'fact', 'billion', 'millions', 'petrol', 'toiletpaperpanic', 'increases', 'steps', 'absolutely', 'ridiculous', 'within', 'areas', 'sense', 'either', 'aisle', 'europe', 'lock', 'shares', 'whether', 'baby', 'wanted', 'bare', 'couldn', 'practice', 'town', 'offers', 'regular', 'often', 'standing', 'fed', 'panicbuyinguk', 'wonder', 'telling', 'station', 'decline', 'patients', 'amidst', 'cough', 'internet', 'created', 'warning', 'air', 'checkout', 'epidemic', 'wants', 'walking', 'include', 'heard', 'slow', 'rights', 'lead', 'causing', 'stocking', 'raising', 'app', 'pressure', 'waste', 'total', 'prepare', 'older', 'maintain', 'respect', 'lady', 'isolate', 'weekend', 'pharmacies', 'appreciate', 'thursday', 'opportunity', 'chance', 'exposed', 'value', 'create', 'arabia', 'fda', 'facebook', 'space', 'busy', 'operations', 'temporary', 'mom', 'lol', 'till', 'common', 'decided', 'waitrose', 'white', 'unless', 'enter', 'add', 'black', 'levels', 'property', 'wish', 'break', 'unable', 'effective', 'narendramodi', 'shipping', 'completely', 'lack', 'minutes', 'governments', 'hunger', 'fraud', 'literally', 'chicken', 'senior', 'happening', 'deserve', 'scared', 'kits', 'commodity', 'options', 'cashiers', 'volunteers', 'felt', 'york', 'super', 'grateful', 'sent', 'uncertainty', 'limits', 'assistance', 'lowest', 'private', 'hike', 'greater', 'largest', 'funny', 'isolating', 'trading', 'feet', 'box', 'wife', 'friday', 'owners', 'focus', 'sanitiser', 'coronaviruslockdown', 'double', 'tax', 'cashier', 'competition', 'brand', 'queues', 'agree', 'novel', 'spreads', 'affect', 'guy', 'cuts', 'charge', 'entire', 'guidelines', 'aware', 'except', 'bag', 'guidance', 'return', 'bottles', 'doctor', 'everyday', 'reported', 'asian', 'bc', 'middle', 'happens', 'fund', 'commission', 'alone', 'feeling', 'target', 'delivering', 'schools', 'transport', 'trips', 'nigeria', 'confirmed', 'reach', 'nearly', 'husband', 'impacting', 'answer', 'profits', 'shifts', 'fully', 'watching', 'sellers', 'driven', 'example', 'worked', 'tech', 'mother', 'supporting', 'play', 'concerned', 'dealing', 'greedy', 'finding', 'matter', 'governor', 'recovery', 'updated', 'fair', 'apparently', 'significant', 'sad', 'beyond', 'traffic', 'everywhere', 'wage', 'manufacturing', 'dairy', 'book', 'purchases', 'industries', 'suppliers', 'threat', 'rates', 'numbers', 'consumption', 'worldwide', 'recently', 'reality', 'sunday', 'stupid', 'leading', 'minimum', 'member', 'infection', 'item', 'piece', 'tv', 'cancelled', 'led', 'coronavirususa', 'leaving', 'activity', 'bus', 'officials', 'inflation', 'forward', 'prepared', 'pre', 'damn', 'shutdown', 'british', 'flour', 'ordered', 'nyc', 'complete', 'pass', 'boost', 'serve', 'dying', 'q', 'pantries', 'allowing', 'received', 'stress', 'study', 'output', 'leaders', 'immediately', 'sa', 'fun', 'ongoing', 'wipe', 'park', 'teachers', 'texas', 'doors', 'cnn', 'h', 'estate', 'eu', 'provided', 'site', 'late', 'solution', 'payment', 'combat', 'dad', 'florida', 'receive', 'additional', 'ventilators', 'catch', 'interest', 'treat', 'road', 'beer', 'fell', 'cover', 'immune', 'game', 'mobile', 'producing', 'moving', 'firms', 'average', 'affairs', 'meeting', 'behaviors', 'sorry', 'problems', 'account', 'creating', 'sharing', 'africa', 'treatment', 'medicine', 'disabled', 'ordering', 'quality', 'payments', 'pmoindia', 'toiletpaperapocalypse', 'perfect', 'ass', 'discuss', 'veg', 'tried', 'corporate', 'precautions', 'farm', 'pickup', 'capacity', 'reporting', 'crash', 'warehouse', 'agriculture', 'mum', 'sending', 'source', 'page', 'hiring', 'cope', 'experiencing', 'dog', 'affecting', 'drugs', 'management', 'address', 'ml', 'toronto', 'six', 'towards', 'clients', 'conditions', 'everybody', 'risking', 'mail', 'brought', 'view', 'analysis', 'negative', 'disinfectant', 'physical', 'hell', 'women', 'aid', 'pls', 'effort', 'idiots', 'claims', 'investors', 'kroger', 'necessities', 'dm', 'fill', 'nobody', 'benefit', 'traders', 'fine', 'australian', 'gift', 'planning', 'socialdistanacing', 'name', 'supposed', 'bills', 'humanity', 'course', 'carry', 'main', 'costco', 'slot', 'west', 'throughout', 'students', 'manage', 'losing', 'smart', 'emails', 'happened', 'international', 'explains', 'loo', 'charging', 'wondering', 'trust', 'zero', 'young', 'manufacturers', 'surrounding', 'professionals', 'feels', 'touching', 'center', 'lockdowns', 'guide', 'heart', 'spent', 'five', 'queuing', 'ft', 'event', 'cart', 'vs', 'series', 'spain', 'pantry', 'sectors', 'attorney', 'platform', 'pet', 'clothes', 'manager', 'gallon', 'son', 'stopstockpiling', 'piersmorgan', 'earlier', 'wouldn', 'wtf', 'virtual', 'percent', 'strategy', 'required', 'thread', 'owner', 'seem', 'limiting', 'population', 'stopped', 'shock', 'men', 'launched', 'official', 'dropping', 'shouldn', 'bringing', 'coronacrisisuk', 'wednesday', 'press', 'meanwhile', 'crowded', 'cars', 'personnel', 'council', 'north', 'child', 'indian', 'cleaners', 'challenge', 'choice', 'pricegouging', 'hits', 'vital', 'photo', 'panicshopping', 'airlines', 'retailer', 'purchasing', 'request', 'tuesday', 'oz', 'shout', 'arrested', 'plastic', 'anywhere', 'spot', 'spike', 'cant', 'affordable', 'expert', 'vaccine', 'helps', 'normally', 'electricity', 'signs', 'im', 'l', 'dollar', 'sainsbury', 'shelter', 'grow', 'hot', 'twitter', 'gives', 'hello', 'survival', 'movement', 'register', 'whilst', 'turning', 'above', 'respond', 'packages', 'charged', 'deep', 'sites', 'central', 'la', 'locations', 'word', 'form', 'crowds', 'puts', 'covidiots', 'reminder', 'coffee', 'staples', 'lysol', 'lose', 'protecting', 'coles', 'class', 'difference', 'flu', 'chaos', 'hire', 'finance', 'frozen', 'process', 'laid', 'picture', 'uncertain', 'looked', 'cooking', 'domestic', 'option', 'surgical', 'pakistan', 'checks', 'germs', 'playing', 'daughter', 'helpful', 'saturday', 'definitely', 'practices', 'providers', 'disgusting', 'totally', 'battle', 'tip', 'exposure', 'perhaps', 'neighbors', 'individuals', 'gave', 'parking', 'tissue', 'concern', 'stuck', 'streets', 'humans', 'packed', 'round', 'stockpile', 'google', 'trend', 'spring', 'loss', 'hungry', 'sweep', 'risks', 'humor', 'saving', 'cards', 'cdc', 'rationing', 'approved', 'includes', 'caught', 'sees', 'wuhan', 'thoughts', 'ticket', 'cities', 'complaints', 'remains', 'tells', 'points', 'safely', 'protections', 'events', 'hiked', 'germany', 'pricing', 'issued', 'responsible', 'curbside', 'stories', 'donated', 'directly', 'advertising', 'natural', 'asia', 'losses', 'meal', 'handle', 'wasn', 'joke', 'environment', 'quite', 'scary', 'rush', 'trolley', 'tough', 'offered', 'nd', 'beware', 'centre', 'flattenthecurve', 'washyourhands', 'hundreds', 'catching', 'rich', 'loans', 'staysafestayhome', 'restock', 'thru', 'ny', 'beans', 'ban', 'holiday', 'wholesale', 'sanitize', 'investment', 'results', 'extremely', 'attention', 'floor', 'logistics', 'technology', 'partner', 'ag', 'onlineshopping', 'funds', 'simply', 'shutting', 'spray', 'capital', 'coughed', 'talks', 'responding', 'hasn', 'proud', 'eye', 'woolworths', 'room', 'usually', 'publix', 'supplychain', 'refund', 'mostly', 'canadian', 'none', 'sports', 'systems', 'medicines', 'petition', 'decision', 'peak', 'wow', 'whatever', 'kenya', 'scientists', 'grab', 'actions', 'struggle', 'ur', 'ontario', 'okay', 'clearly', 'holding', 'prime', 'selfisolation', 'quarantined', 'pubs', 'mall', 'agreed', 'apply', 'causes', 'mouth', 'dangerous', 'enjoy', 'added', 'search', 'suffering', 'silver', 'chicago', 'basket', 'solutions', 'barrel', 'content', 'beef', 'reducing', 'episode', 'type', 'ill', 'model', 'britain', 'au', 'truly', 'miss', 'apps', 'encourage', 'knew', 'european', 'expectations', 'auspol', 'challenging', 'rapidly', 'ration', 'expensive', 'towels', 'immediate', 'began', 'patterns', 'outlook', 'flight', 'wide', 'considering', 'february', 'track', 'apocalypse', 'revenue', 'building', 'anti', 'greed', 'heading', 'apple', 'managing', 'exercise', 'claim', 'sanitizing', 'leadership', 'disaster', 'resource', 'facilities', 'discount', 'regularly', 'organizations', 'otherwise', 'posted', 'nose', 'glad', 'words', 'begin', 'turned', 'canned', 'provides', 'illegal', 'released', 'announcement', 'particularly', 'managed', 'ground', 'bars', 'hazard', 'charity', 'contain', 'unfortunately', 'yeah', 'mortgage', 'considered', 'faces', 'raised', 'joe', 'ends', 'pray', 'highly', 'climate', 'fees', 'opened', 'storm', 'agencies', 'surely', 'proper', 'capitalism', 'piling', 'statement', 'com', 'season', 'handling', 'loved', 'file', 'tracking', 'letter', 'program', 'shifting', 'knows', 'tweet', 'avoiding', 'chief', 'cents', 'weird', 'fixed', 'ministry', 'visiting', 'continued', 'unnecessary', 'warned', 'weather', 'france', 'stations', 'de', 'keeps', 'carts', 'severe', 'boris', 'significantly', 'starts', 'spoke', 'kept', 'kindly', 'download', 'wages', 'task', 'disruption', 'patient', 'bbc', 'rd', 'exorbitant', 'january', 'dedicated', 'bottom', 'v', 'cold', 'fellow', 'dry', 'absolute', 'popular', 'becoming', 'executive', 'donation', 'vaccines', 'killing', 'sister', 'packs', 'driver', 'ply', 'deals', 'pa', 'passed', 'awareness', 'beat', 'learning', 'multiple', 'fruits', 'adapt', 'network', 'surfaces', 'gel', 'education', 'availability', 'win', 'authorities', 'ship', 'loan', 'rely', 'administration', 'sky', 'surging', 'cannabis', 'bay', 'warns', 'sharp', 'eyes', 'afraid', 'friendly', 'impossible', 'majority', 'reading', 'anyway', 'safer', 'text', 'useful', 'urgent', 'helped', 'hearing', 'flights', 'rule', 'actual', 'walked', 'boxes', 'podcast', 'canadians', 'freeze', 'coronapocolypse', 'games', 'green', 'measure', 'commercial', 'director', 'washington', 'photos', 'music', 'minute', 'deadly', 'curfew', 'cool', 'trolleys', 'fish', 'corner', 'chinesevirus', 'push', 'remote', 'runs', 'writes', 'forcing', 'possibly', 'san', 'animal', 'role', 'careful', 'aldi', 'postal', 'east', 'plant', 'stockup', 'diy', 'noticed', 'student', 'kitchen', 'ya', 'closure', 'homemade', 'shared', 'ocado', 'indoors', 'speak', 'prevention', 'thankyou', 'section', 'ads', 'visits', 'bbcnews', 'pictures', 'quaratinelife', 'japan', 'auto', 'jump', 'red', 'videos', 'trader', 'kit', 'truckers', 'mar', 'nationwide', 'welcome', 'earth', 'picking', 'shall', 'mine', 'kills', 'movie', 'pushing', 'civil', 'dead', 'cutting', 'vegetable', 'mad', 'plunge', 'congress', 'fire', 'insight', 'laws', 'hospitality', 'build', 'bekind', 'dies', 'hopefully', 'contracting', 'collect', 'curb', 'luxury', 'doubled', 'regulations', 'grocers', 'drops', 'wave', 'reasons', 'authority', 'policies', 'thankful', 'history', 'union', 'gasoline', 'extreme', 'summer', 'johnson', 'viruses', 'bonus', 'paracetamol', 'straight', 'nj', 'opportunities', 'clerk', 'mr', 'forever', 'protected', 'michigan', 'utility', 'beauty', 'drink', 'practicing', 'evidence', 'counter', 'blame', 'easily', 'curve', 'reasonable', 'budget', 'tackle', 'cent', 'rural', 'urge', 'stepping', 'sanitation', 'ireland', 'ups', 'dc', 'stable', 'lockdownuk', 'various', 'continuing', 'executives', 'informed', 'focused', 'pub', 'interview', 'extended', 'partners', 'fox', 'sudden', 'delayed', 'trouble', 'index', 'sharply', 'developed', 'gop', 'shelterinplace', 'toward', 'sanitisers', 'alive', 'brother', 'entering', 'toll', 'transmission', 'kg', 'rapid', 'posting', 'firm', 'gear', 'groceryshopping', 'laugh', 'independent', 'workforce', 'operators', 'adding', 'failed', 'body', 'slowdown', 'links', 'church', 'pop', 'answers', 'cancel', 'hotels', 'sitting', 'held', 'range', 'potentially', 'distribute', 'hul', 'party', 'suffer', 'nz', 'serving', 'teams', 'although', 'shopper', 'abc', 'wanna', 'inventory', 'bar', 'madness', 'closes', 'liquor', 'art', 'fashion', 'opinion', 'interested', 'boss', 'acting', 'filling', 'army', 'suddenly', 'brilliant', 'foreign', 'exactly', 'lt', 'warn', 'cov', 'isolated', 'coping', 'ca', 'filled', 'complaint', 'excited', 'convenience', 'announces', 'evening', 'asset', 'iran', 'killed', 'region', 'highest', 'funding', 'midst', 'homeless', 'star', 'singapore', 'hopes', 'colleagues', 'officers', 'entertainment', 'egg', 'reduction', 'excessive', 'specific', 'choose', 'aldiuk', 'compared', 'release', 'university', 'netflix', 'enforcement', 'malaysia', 'third', 'location', 'ashamed', 'ad', 'mark', 'falls', 'club', 'sometimes', 'bloody', 'coronavirusindia', 'terms', 'creative', 'youtube', 'tears', 'coronaviruschallenge', 'bet', 'hero', 'marketers', 'bored', 'ease', 'responses', 'whose', 'charities', 'cat', 'desperate', 'activities', 'reveals', 'visited', 'similar', 'discusses', 'becomes', 'allegedly', 'dramatic', 'min', 'ice', 'disinfecting', 'ain', 'cheaper', 'tourism', 'legal', 'college', 'cheese', 'era', 'hate', 'slash', 'faster', 'professor', 'basically', 'covering', 'malls', 'gratitude', 'disruptions', 'economies', 'realize', 'learned', 'awesome', 'hair', 'globally', 'published', 'contract', 'soar', 'turns', 'guns', 'employment', 'adults', 'programs', 'urges', 'distillery', 'explain', 'hub', 'exploiting', 'knowing', 'hr', 'platforms', 'approach', 'plummeting', 'pharmacists', 'rental', 'forces', 'smaller', 'sort', 'exports', 'labor', 'finds', 'disinfect', 'crowd', 'session', 'excuse', 'plummet', 'attitudes', 'bleach', 'corn', 'gathering', 'covidiot', 'corvid', 'basis', 'households', 'carrying', 'pump', 'checking', 'skyrocketing', 'putin', 'suggest', 'comfort', 'taxes', 'drives', 'guard', 'code', 'charges', 'collection', 'skyrocket', 'scare', 'nations', 'cdcgov', 'stayhomechallenge', 'sugar', 'manchester', 'operating', 'transportation', 'channel', 'russian', 'plunging', 'futures', 'assist', 'uses', 'demands', 'parks', 'inflating', 'alternative', 'panicking', 'extortionate', 'hoping', 'websites', 'preparing', 'easier', 'dinner', 'mid', 'known', 'potatoes', 'harder', 'finances', 'urged', 'seconds', 'managers', 'letting', 'political', 'slump', 'carers', 'retweet', 'buys', 'monthly', 'itself', 'lets', 'volunteer', 'ability', 'association', 'los', 'afternoon', 'priorities', 'phishing', 'vote', 'uber', 'cook', 'dramatically', 'window', 'purchased', 'mission', 'announce', 'lows', 'coverage', 'economics', 'damage', 'gain', 'warehouses', 'refusing', 'switch', 'dollars', 'touched', 'parts', 'throw', 'stripped', 'movies', 'selfishness', 'german', 'facemask', 'bitcoin', 'board', 'stopthespread', 'devices', 'litre', 'diesel', 'saudiarabia', 'frequently', 'heb', 'navigate', 'existing', 'agricultural', 'king', 'launches', 'fewer', 'corporations', 'contest', 'anxious', 'treatments', 'understanding', 'ethanol', 'idiot', 'vendors', 'science', 'accounts', 'oott', 'smile', 'surprise', 'honestly', 'military', 'circumstances', 'figure', 'placed', 'moved', 'mckinsey', 'cos', 'cross', 'distilleries', 'stayhomestaysafe', 'lucky', 'trillion', 'ingredients', 'agency', 'condition', 'implications', 'recover', 'nofood', 'insane', 'un', 'trending', 'lately', 'briefing', 'categories', 'export', 'arrived', 'present', 'listed', 'basics', 'convid', 'importance', 'ceos', 'picked', 'books', 'reaching', 'usd', 'amounts', 'uklockdown', 'maga', 'potus', 'cattle', 'stopping', 'skynews', 'anymore', 'wrote', 'analyst', 'analysts', 'banking', 'lagos', 'carriers', 'fallout', 'coronavirusupdates', 'monitor', 'plz', 'campaign', 'plants', 'depression', 'factories', 'soup', 'gotta', 'responsibility', 'houses', 'scale', 'kindness', 'false', 'globe', 'animals', 'bn', 'missing', 'surprised', 'mode', 'realestate', 'covered', 'gun', 'thinks', 'standards', 'jack', 'monitoring', 'border', 'turkey', 'lining', 'position', 'suspend', 'morons', 'strict', 'paisley', 'cbc', 'metro', 'edition', 'garden', 'nygovcuomo', 'decisions', 'wheat', 'bacteria', 'broke', 'entry', 'mention', 'shutdowns', 'ran', 'crying', 'rose', 'bog', 'perspective', 'taste', 'gdp', 'messages', 'table', 'fridge', 'ghana', 'bankers', 'psa', 'appreciated', 'makers', 'neighborhood', 'remind', 'infections', 'economist', 'committed', 'crucial', 'icymi', 'hoax', 'hurt', 'takeout', 'doubt', 'maintaining', 'baskets', 'veggies', 'shown', 'nor', 'facemasks', 'coughs', 'dump', 'laundry', 'meme', 'viral', 'privacy', 'hrs', 'favorite', 'pizza', 'safeway', 'founder', 'scarce', 'wiped', 'fix', 'booming', 'individual', 'packaging', 'memes', 'yourselves', 'introduced', 'appears', 'stops', 'holidays', 'danger', 'initiative', 'innovation', 'snap', 'willing', 'overall', 'minimize', 'forecast', 'conference', 'certainly', 'bunch', 'raw', 'hotline', 'pennsylvania', 'express', 'whatsapp', 'count', 'brexit', 'nature', 'african', 'attack', 'load', 'final', 'slowing', 'sleep', 'bid', 'prior', 'western', 'chinavirus', 'french', 'comedy', 'distributors', 'freezer', 'stick', 'phase', 'sit', 'strange', 'experiences', 'seattle', 'failing', 'accept', 'lovely', 'tired', 'wonderful', 'abuse', 'aside', 'cream', 'wall', 'players', 'neighbours', 'reached', 'merchants', 'mental', 'et', 'permanent', 'addition', 'associates', 'previously', 'receiving', 'grocerystores', 'industrial', 'field', 'christmas', 'metres', 'victim', 'appreciation', 'yours', 'tickets', 'jersey', 'grocerystore', 'pets', 'ideas', 'drinking', 'refunds', 'john', 'steel', 'delay', 'houston', 'cleared', 'unsung', 'base', 'induced', 'garbage', 'surplus', 'england', 'project', 'breaks', 'reusable', 'illness', 'meaning', 'sneeze', 'locked', 'strain', 'poverty', 'al', 'loads', 'hoarded', 'facts', 'types', 'irresponsible', 'conversation', 'yo', 'chocolate', 'dubai', 'strategies', 'earnings', 'paycheck', 'precaution', 'decrease', 'rn', 'begun', 'centers', 'sydney', 'chloroquine', 'gotten', 'joined', 'surges', 'mins', 'ourselves', 'upon', 'usage', 'sun', 'soaring', 'constantly', 'cures', 'atm', 'girl', 'launch', 'crises', 'included', 'foot', 'lab', 'sadly', 'angry', 'invest', 'brad', 'steal', 'closer', 'refuse', 'flat', 'sensible', 'feeding', 'flying', 'claiming', 'streaming', 'poll', 'connect', 'democrats', 'stressful', 'trash', 'immunity', 'implement', 'previous', 'governors', 'storage', 'pork', 'secure', 'fallen', 'beverage', 'transit', 'users', 'rock', 'resilient', 'former', 'halt', 'trucks', 'blow', 'handwash', 'properly', 'officer', 'md', 'fever', 'brings', 'banned', 'exchange', 'lift', 'lunch', 'meant', 'responsibly', 'overnight', 'shoes', 'factory', 'staple', 'panicbuyers', 'version', 'evolving', 'uae', 'petroleum', 'sucks', 'ssupnow', 'bureau', 'fightcovid', 'operate', 'planet', 'clothing', 'valuable', 'dirty', 'cancellations', 'northern', 'valley', 'hitting', 'resulting', 'nytimes', 'covididiots', 'wiping', 'blue', 'suspect', 'leader', 'korea', 'kid', 'mumbai', 'aus', 'lidlgb', 'classes', 'emptying', 'inc', 'underlying', 'fmcg', 'j', 'duty', 'tank', 'restricted', 'widespread', 'threats', 'planned', 'purpose', 'quarter', 'setting', 'tea', 'perishable', 'obviously', 'investing', 'formula', 'bless', 'particular', 'posts', 'foodbanks', 'accelerate', 'max', 'materials', 'lidl', 'besides', 'hardest', 'senate', 'increasingly', 'yr', 'unemployed', 'starve', 'forbes', 'luck', 'discussion', 'advised', 'drinks', 'cbd', 'recipe', 'june', 'experienced', 'leads', 'rip', 'vouchers', 'fiscal', 'disposable', 'bailout', 'caring', 'discounts', 'pro', 'gatherings', 'expects', 'surface', 'heavily', 'hardship', 'village', 'fraudulent', 'listening', 'speed', 'insecurity', 'delays', 'reuters', 'combined', 'stressed', 'seek', 'slowly', 'weak', 'reaction', 'wholefoods', 'nowhere', 'blood', 'dumping', 'savings', 'comment', 'nigerians', 'exclusive', 'highlights', 'restocking', 'donald', 'projects', 'became', 'stupidity', 'comments', 'construction', 'alberta', 'excellent', 'attempt', 'downturn', 'layoffs', 'spirit', 'onto', 'twice', 'enforce', 'volume', 'politicians', 'shot', 'checked', 'urging', 'equity', 'initial', 'minnesota', 'standard', 'soaps', 'size', 'marketplace', 'shameful', 'firefighters', 'machine', 'fl', 'cares', 'quarentinelife', 'imposed', 'met', 'imports', 'reserve', 'feb', 'dining', 'butter', 'pushed', 'currency', 'slashed', 'scenes', 'steep', 'mayor', 'drastically', 'suspended', 'designed', 'incredible', 'papers', 'boom', 'wild', 'ignore', 'spitting', 'answered', 'bin', 'packaged', 'beautiful', 'foodbank', 'spare', 'tools', 'clearing', 'land', 'destroy', 'review', 'purell', 'dream', 'dogs', 'anybody', 'clorox', 'pr', 'collectors', 'radio', 'development', 'puzzle', 'calculator', 'worries', 'iraq', 'heartbreaking', 'volatility', 'cc', 'lowering', 'employers', 'horrible', 'clock', 'heavy', 'italian', 'lie', 'units', 'nielsen', 'developing', 'exploit', 'ai', 'miles', 'criminal', 'faith', 'antibacterial', 'explore', 'vigilant', 'training', 'denmark', 'missed', 'clubs', 'asks', 'booked', 'truth', 'gym', 'highlight', 'mandatory', 'predict', 'treated', 'lenders', 'lessons', 'assholes', 'direction', 'recipes', 'fault', 'dark', 'brave', 'reflect', 'wasted', 'ending', 'sells', 'realise', 'unilever', 'oilprice', 'district', 'sounds', 'lies', 'passes', 'opens', 'ohio', 'inflate', 'lockdowneffect', 'fit', 'ten', 'nursing', 'returns', 'welfare', 'persons', 'dozen', 'financially', 'illinois', 'emerging', 'suggests', 'connected', 'indiafightscorona', 'pull', 'channels', 'assets', 'demanding', 'signed', 'sources', 'bidet', 'communications', 'sound', 'finished', 'sars', 'writing', 'mini', 'dates', 'en', 'kinda', 'adapting', 'beach', 'downingstreet', 'dow', 'develop', 'closely', 'charmin', 'produced', 'somebody', 'asymptomatic', 'legislation', 'tons', 'trick', 'appear', 'colorado', 'pharmaceutical', 'postponed', 'seller', 'borders', 'shirt', 'temperature', 'wet', 'hysteria', 'react', 'skyrocketed', 'stayathomechallenge', 'dip', 'voice', 'comprehensive', 'processing', 'correct', 'dude', 'fb', 'hypermarket', 'birthday', 'chat', 'chances', 'chemicals', 'cats', 'michael', 'asap', 'shocking', 'treating', 'emptied', 'lending', 'closings', 'eligible', 'headed', 'rises', 'bigger', 'hang', 'suppose', 'provider', 'promise', 'sight', 'officially', 'shocked', 'thrown', 'implemented', 'citizen', 'crashing', 'opposite', 'cleaner', 'handles', 'crap', 'inevitable', 'stepped', 'faced', 'airline', 'starvation', 'ph', 'tho', 'entrance', 'frontlines', 'workfromhome', 'collapsing', 'na', 'expecting', 'groceryworkers', 'op', 'design', 'recommend', 'pace', 'routine', 'toiletries', 'prepper', 'unit', 'performance', 'prove', 'rude', 'morrison', 'tracker', 'tenants', 'needing', 'disinfectants', 'tinned', 'practical', 'meds', 'appeal', 'disappointed', 'licking', 'hikes', 'bitch', 'screen', 'mitigate', 'examples', 'employed', 'mexico', 'historic', 'meter', 'arizona', 'jesus', 'lowered', 'sufficient', 'recommendations', 'requires', 'gap', 'giveawayalert', 'offices', 'diet', 'status', 'category', 'bathroom', 'province', 'david', 'angeles', 'vancouver', 'adjusting', 'unique', 'grade', 'infrastructure', 'chill', 'division', 'lesson', 'instacart', 'needy', 'massachusetts', 'active', 'operation', 'roof', 'oversupply', 'considerate', 'declining', 'airport', 'pause', 'unlikely', 'bastards', 'maximum', 'constant', 'ensuring', 'guardian', 'boycott', 'earn', 'actors', 'socially', 'convenient', 'medicare', 'fraudsters', 'prefer', 'distributed', 'gavinnewsom', 'thats', 'flowers', 'poultry', 'walks', 'coronaoutbreak', 'engage', 'heads', 'ended', 'vegan', 'electric', 'gmb', 'spikes', 'brick', 'tune', 'australians', 'transactions', 'adequate', 'ignoring', 'famous', 'discounted', 'owned', 'diagnosed', 'discover', 'excess', 'proof', 'switching', 'scheduled', 'sundaythoughts', 'foxnews', 'contribute', 'effectively', 'flipkart', 'jumped', 'producer', 'electronics', 'consequences', 'awful', 'pocket', 'jacking', 'canon', 'psychology', 'father', 'reserves', 'researchers', 'ma', 'panel', 'zealand', 'unexpected', 'respiratory', 'fail', 'coronaviruslockdownuk', 'coronapocalypse', 'involved', 'stage', 'farms', 'promote', 'rescue', 'fee', 'scarcity', 'stripping', 'tiktok', 'passing', 'teens', 'potato', 'prescription', 'selfquarantine', 'overwhelming', 'ammo', 'bans', 'zoom', 'passengers', 'smh', 'cnbc', 'sh', 'turbo', 'locally', 'oman', 'stayhealthy', 'deliberately', 'meters', 'write', 'bakery', 'utilities', 'web', 'indeed', 'relevant', 'evil', 'miami', 'mart', 'ramping', 'soars', 'panicked', 'steady', 'freedom', 'hotel', 'improve', 'rushing', 'mess', 'janitors', 'freezers', 'medications', 'cans', 'joining', 'respirators', 'controlled', 'pics', 'flow', 'wore', 'ffs', 'breathing', 'panickbuying', 'spirits', 'declined', 'kong', 'threatening', 'wfh', 'broken', 'quantities', 'solidarity', 'juice', 'unlike', 'schedule', 'da', 'instructions', 'require', 'skin', 'toiletpaperemergency', 'roads', 'neighbour', 'attendants', 'behaviours', 'hunt', 'organisations', 'modi', 'multi', 'watched', 'matthancock', 'tool', 'complaining', 'spotted', 'crore', 'relying', 'saved', 'encouraging', 'balance', 'desperation', 'granted', 'yall', 'urgently', 'suggestions', 'stealing', 'org', 'profiting', 'contactless', 'handy', 'advocates', 'preventing', 'salary', 'seafood', 'sarscov', 'returned', 'coronavirusaustralia', 'pile', 'plummeted', 'organization', 'nervous', 'justice', 'nearest', 'chemist', 'winning', 'largely', 'ride', 'reporter', 'nba', 'style', 'realized', 'contracted', 'race', 'takeaway', 'somewhere', 'tissues', 'accused', 'triple', 'victims', 'tx', 'farming', 'regional', 'obvious', 'nhsuk', 'tirelessly', 'emptyshelves', 'therefore', 'ramp', 'cuomo', 'crime', 'unfair', 'whenever', 'secretary', 'precautionary', 'foundation', 'sake', 'built', 'contracts', 'success', 'handwashing', 'premier', 'investments', 'outrageous', 'hindustan', 'pharma', 'failure', 'train', 'observe', 'encouraged', 'southafrica', 'matters', 'contamination', 'cuz', 'guards', 'coronavirusoutbreakindia', 'genius', 'bio', 'cloth', 'neighbor', 'coronavid', 'gallons', 'image', 'upcoming', 'barely', 'wealth', 'shifted', 'collective', 'pic', 'begins', 'hong', 'folk', 'socialdistance', 'disrupted', 'ie', 'lying', 'uganda', 'republicans', 'plague', 'asthma', 'extend', 'whoever', 'eventually', 'notes', 'flatten', 'listings', 'socialism', 'sneezing', 'election', 'genuinely', 'court', 'towel', 'showed', 'exhausted', 'profiteers', 'farmer', 'cpg', 'peace', 'contributing', 'preparation', 'shape', 'bullshit', 'btw', 'dept', 'msnbc', 'distributing', 'oilprices', 'exposing', 'gather', 'regulatory', 'culture', 'annual', 'badly', 'lasting', 'cdnpoli', 'terrible', 'medication', 'maryland', 'drastic', 'stockpilinguk', 'stayathomesavelives', 'communication', 'arrest', 'appropriate', 'commissioner', 'nearby', 'fields', 'scenario', 'nashville', 'snacks', 'quiet', 'unrest', 'risen', 'raises', 'figures', 'venture', 'elsewhere', 'personally', 'stockmarket', 'remove', 'allows', 'behave', 'wisconsin', 'confused', 'soft', 'fyi', 'cap', 'speaks', 'analytics', 'recommends', 'agreement', 'gasprices', 'stronger', 'dealers', 'golf', 'infect', 'esp', 'football', 'theres', 'fined', 'honest', 'bubble', 'plea', 'whitehouse', 'salute', 'essentialworkers', 'forgot', 'coast', 'mood', 'overtime', 'traditional', 'adjust', 'incomes', 'outlets', 'dumb', 'breath', 'predicted', 'canceled', 'suggested', 'powerful', 'restocked', 'mile', 'thailand', 'declines', 'rally', 'mentioned', 'moves', 'zone', 'carbon', 'vehicles', 'linked', 'shoot', 'crypto', 'fines', 'neither', 'qatar', 'peoples', 'gains', 'strongly', 'id', 'utter', 'cake', 'surged', 'takeaways', 'medium', 'screens', 'politics', 'mixed', 'accepting', 'zombie', 'grows', 'assume', 'arrive', 'automotive', 'chart', 'views', 'thermometers', 'curious', 'shoprite', 'giants', 'complain', 'quit', 'bright', 'wise', 'crop', 'isle', 'bushcraft', 'hosting', 'slightly', 'worrying', 'liquidity', 'brooklyn', 'lng', 'severely', 'decide', 'factors', 'mainly', 'attacks', 'diseases', 'stockpiled', 'norm', 'lists', 'teenagers', 'original', 'proven', 'newsletter', 'scene', 'coupled', 'generation', 'bailouts', 'landlords', 'trumpvirus', 'witnessed', 'ignorant', 'fights', 'merkel', 'shopkeepers', 'commissions', 'reopen', 'gluten', 'recommended', 'buyer', 'rid', 'orange', 'scott', 'bidding', 'seeds', 'sweet', 'oilpricewar', 'designated', 'racism', 'toiletpaperchallenge', 'decent', 'importantly', 'tills', 'misinformation', 'handed', 'impose', 'estimated', 'investigation', 'sat', 'crashed', 'assure', 'biz', 'repost', 'dutch', 'fao', 'sanctions', 'collapsed', 'terrified', 'battling', 'compromised', 'identified', 'lied', 'las', 'remaining', 'fixing', 'returning', 'keyworkers', 'leaves', 'advise', 'applied', 'wholesalers', 'incredibly', 'knowledge', 'danish', 'nutrition', 'bargain', 'sink', 'mining', 'expose', 'ex', 'employer', 'universal', 'coronavirusmemes', 'hurts', 'noon', 'findings', 'labour', 'affects', 'forgotten', 'strategic', 'attend', 'situations', 'thus', 'booze', 'emotional', 'professional', 'associated', 'uptick', 'prison', 'liquid', 'machines', 'parent', 'depend', 'snapshot', 'software', 'ambulance', 'mp', 'announcing', 'supplying', 'values', 'landscape', 'supported', 'stamps', 'pleased', 'choices', 'revenues', 'actively', 'mortar', 'nope', 'modern', 'filed', 'followed', 'operational', 'flies', 'plunged', 'supplier', 'devastating', 'workingfromhome', 'brown', 'film', 'institutions', 'coronavirusaus', 'stockers', 'wealthy', 'surviving', 'outbreaks', 'supplied', 'throwing', 'phones', 'senator', 'dozens', 'permanently', 'iceland', 'parties', 'shocks', 'rubbing', 'hill', 'godrej', 'priced', 'preferences', 'schemes', 'childcare', 'ton', 'uv', 'lettuce', 'finish', 'organic', 'ke', 'tiny', 'artists', 'dallas', 'toiletroll', 'metal', 'tokyo', 'tag', 'commitment', 'recorded', 'enable', 'corp', 'client', 'maker', 'stolen', 'wsj', 'doorstep', 'lived', 'slashing', 'rob', 'pulling', 'daylockdown', 'bbb', 'labs', 'random', 'airborne', 'pc', 'tariffs', 'shoplocal', 'square', 'boy', 'riots', 'qualify', 'committee', 'couples', 'shower', 'somehow', 'em', 'deck', 'amongst', 'facility', 'locals', 'shield', 'budgets', 'stood', 'bs', 'cute', 'grip', 'releases', 'vegas', 'sam', 'institute', 'acts', 'que', 'weren', 'parcels', 'portal', 'joy', 'host', 'francisco', 'suit', 'triggered', 'handing', 'cleaned', 'wars', 'solve', 'disgraceful', 'pieces', 'downward', 'spaces', 'cancellation', 'drone', 'warnings', 'harvest', 'rebound', 'rough', 'gb', 'song', 'fans', 'diabetes', 'stackers', 'boston', 'brent', 'seven', 'mix', 'complex', 'expanded', 'confirms', 'icu', 'startup', 'calpol', 'besafe', 'aim', 'overcome', 'shipments', 'baking', 'ward', 'premium', 'delhi', 'severity', 'overseas', 'regime', 'ps', 'talked', 'unnecessarily', 'containment', 'assured', 'material', 'habit', 'ikea', 'paramedics', 'bosses', 'bottled', 'tom', 'hollywood', 'wti', 'ccp', 'clue', 'trained', 'harm', 'freaking', 'tweets', 'omg', 'abt', 'cry', 'powers', 'useless', 'boosting', 'rooms', 'nigerian', 'jeff', 'wells', 'cell', 'icis', 'island', 'instagram', 'rations', 'deemed', 'adult', 'declared', 'prank', 'pure', 'computer', 'james', 'preparedness', 'wuhanvirus', 'volatile', 'cupboards', 'discussing', 'reveal', 'permitted', 'identify', 'postpone', 'fastest', 'intentionally', 'barrels', 'richard', 'removed', 'fabric', 'jones', 'carrier', 'searching', 'rare', 'repeat', 'courtesy', 'covi', 'pain', 'se', 'apr', 'chemical', 'intelligence', 'armed', 'refused', 'el', 'blocking', 'le', 'ford', 'corrupt', 'baked', 'dan', 'govmurphy', 'recovered', 'van', 'chairman', 'gamestop', 'despicable', 'discovered', 'decade', 'kenyans', 'prayers', 'loaf', 'cyber', 'cruise', 'george', 'scheme', 'collected', 'wont', 'babies', 'capped', 'combination', 'youth', 'ap', 'joins', 'noodles', 'reacting', 'agents', 'looting', 'moratorium', 'overwhelmed', 'hubs', 'finland', 'dive', 'print', 'rubber', 'imported', 'pound', 'texts', 'stabilize', 'saudis', 'hardware', 'remotely', 'focusing', 'inform', 'greatly', 'registers', 'urban', 'bail', 'locusts', 'manufacturer', 'consume', 'requirements', 'relating', 'justintrudeau', 'greatest', 'sticks', 'sub', 'featuring', 'sparks', 'likes', 'strike', 'starbucks', 'generally', 'route', 'senators', 'implementing', 'possibility', 'jail', 'physicaldistancing', 'emerge', 'followers', 'hedge', 'defense', 'tap', 'resist', 'odd', 'oregon', 'stability', 'joint', 'responded', 'eats', 'accurate', 'advance', 'bear', 'written', 'sea', 'crops', 'tweeted', 'lockdownnow', 'connection', 'bankruptcy', 'upset', 'breweries', 'infectious', 'downtown', 'entered', 'copper', 'hole', 'images', 'telehealth', 'resulted', 'speakerpelosi', 'booking', 'relax', 'correction', 'destruction', 'billions', 'shoutout', 'depressed', 'mo', 'yoy', 'coronavirusnewyork', 'fridaythoughts', 'havoc', 'articles', 'headlines', 'bounce', 'proposed', 'praise', 'pledges', 'tumble', 'disgusted', 'centres', 'fema', 'austin', 'foodsecurity', 'manufacture', 'larger', 'expand', 'quarantinediaries', 'branch', 'approve', 'prop', 'hardly', 'racist', 'comply', 'reply', 'sheets', 'revealed', 'regulate', 'stayed', 'pregnant', 'boys', 'digitalmarketing', 'enforcing', 'enforced', 'expenses', 'allergies', 'speaking', 'ignored', 'regards', 'bust', 'vp', 'pair', 'telephone', 'telegraph', 'thanked', 'grown', 'consideration', 'eight', 'trigger', 'spanish', 'specifically', 'apartment', 'immunocompromised', 'statewide', 'fascinating', 'decades', 'cancer', 'wellness', 'hardships', 'crowding', 'creates', 'threatens', 'housewares', 'exist', 'kicked', 'bcg', 'z', 'risky', 'publichealth', 'partnership', 'tape', 'classified', 'sparked', 'strength', 'decreased', 'launching', 'californians', 'fantastic', 'jokes', 'attempting', 'banning', 'pounds', 'suits', 'birx', 'adjusted', 'elections', 'hyderabad', 'jobless', 'prep', 'guarantee', 'eastern', 'block', 'listing', 'contaminated', 'nonprofit', 'introducing', 'cautious', 'residential', 'dress', 'baker', 'associate', 'boredom', 'territory', 'ar', 'primary', 'iphone', 'bogus', 'wanting', 'lifted', 'edge', 'corruption', 'missouri', 'masses', 'virtually', 'jan', 'skills', 'map', 'pumps', 'towns', 'highs', 'paul', 'combating', 'contacted', 'strangers', 'row', 'insurers', 'toys', 'contains', 'stays', 'proceeds', 'necessity', 'vodafone', 'nonsense', 'relatively', 'anticipate', 'breakfast', 'hall', 'eg', 'sets', 'instant', 'tearful', 'reliance', 'smell', 'chemists', 'breakdown', 'dey', 'ultimate', 'rona', 'hydroxychloroquine', 'allah', 'drharshvardhan', 'hurting', 'alternatives', 'ventilator', 'counters', 'successful', 'survived', 'minds', 'himself', 'concept', 'grains', 'speech', 'endless', 'offset', 'ladies', 'scotland', 'packet', 'aerosol', 'favourite', 'frenzy', 'additive', 'pandemics', 'vehicle', 'kantar', 'roughly', 'selfisolating', 'droplets', 'moments', 'packets', 'endure', 'homeworld', 'watchdog', 'pulse', 'struck', 'cashapp', 'marksandspencer', 'trains', 'rents', 'popped', 'involving', 'millennials', 'perfume', 'letters', 'procedures', 'temperatures', 'kudos', 'georgia', 'voting', 'cloud', 'temp', 'suspension', 'voucher', 'signing', 'procurement', 'compare', 'aloe', 'telecom', 'prompted', 'stuffs', 'hunting', 'covidcanada', 'burden', 'confirm', 'fishing', 'corporation', 'patience', 'karma', 'cartoon', 'neighbourhood', 'paranoid', 'dawn', 'lottery', 'aftermath', 'loading', 'thrive', 'highlighting', 'shopped', 'coordinator', 'empathy', 'wasting', 'indoor', 'played', 'statistics', 'tripled', 'misleading', 'londonlockdown', 'hoarder', 'metals', 'celebrities', 'celebrate', 'disrupt', 'slashes', 'silly', 'fuels', 'virginia', 'blessed', 'precious', 'semi', 'salt', 'attitude', 'struggles', 'garage', 'depot', 'dividend', 'mortgages', 'whom', 'sauce', 'midnight', 'donates', 'teach', 'laughing', 'innovative', 'deposit', 'bjp', 'hurry', 'ed', 'behaving', 'exciting', 'kingdom', 'kr', 'pays', 'environmental', 'hadn', 'spit', 'studies', 'peter', 'wellbeing', 'capitalist', 'topics', 'targeted', 'thermometer', 'introduce', 'restriction', 'subscription', 'bonds', 'coronav', 'net', 'reserved', 'quarantineandchill', 'fiji', 'mike', 'requests', 'hide', 'lifeline', 'proposal', 'indefinitely', 'compete', 'methods', 'shuttered', 'enemy', 'unbelievable', 'cx', 'applies', 'glasses', 'summary', 'prey', 'covers', 'contagion', 'criminals', 'furniture', 'surveys', 'weight', 'conducted', 'names', 'knock', 'paint', 'lowe', 'suspending', 'winter', 'passover', 'asshole', 'iowa', 'lay', 'extending', 'melbourne', 'numerous', 'toiletpapergate', 'bell', 'rwanda', 'wedding', 'robust', 'royal', 'scrubs', 'poop', 'coz', 'comics', 'mindset', 'est', 'shipment', 'topic', 'submit', 'accessible', 'firearms', 'restrict', 'guaranteed', 'lingers', 'caps', 'singing', 'buses', 'custom', 'reduces', 'arms', 'cycle', 'provision', 'economists', 'dance', 'seeking', 'usda', 'flexible', 'barriers', 'inthistogether', 'extends', 'bed', 'containers', 'finger', 'brits', 'explained', 'recognize', 'promised', 'estimates', 'metre', 'provisions', 'herd', 'traveling', 'exceed', 'pollution', 'dust', 'ultimately', 'doubling', 'entirely', 'eliminate', 'humble', 'junk', 'oklahoma', 'lockdownuknow', 'tactics', 'ug', 'intended', 'bike', 'seemed', 'israel', 'interact', 'regardless', 'taxi', 'destroyed', 'suggestion', 'foodshortages', 'queueing', 'issuing', 'influence', 'fauci', 'grandparents', 'investigate', 'physically', 'observed', 'starving', 'gate', 'achieve', 'peeps', 'reportedly', 'properties', 'vitamin', 'safeguard', 'zimbabwe', 'controls', 'skip', 'disability', 'follows', 'ignorance', 'chips', 'con', 'fingers', 'vodafoneuk', 'remained', 'regions', 'reminds', 'angela', 'guilty', 'quote', 'venezuela', 'compensation', 'sanitary', 'investor', 'match', 'camera', 'mondaymotivation', 'delicious', 'cheers', 'albertsons', 'publicly', 'unavailable', 'menu', 'requesting', 'shields', 'coal', 'wherever', 'foodstuffs', 'spar', 'bonuses', 'enhanced', 'strictly', 'skyuk', 'hygienic', 'crudeoil', 'roundtable', 'aka', 'vacation', 'atlanta', 'sacrifice', 'transfer', 'wegmans', 'difficulties', 'kagutamuseveni', 'tim', 'societies', 'atleast', 'tins', 'stacking', 'vast', 'scrambling', 'plexiglass', 'fcc', 'vermont', 'andrew', 'competitive', 'journalists', 'soldiers', 'misery', 'helpers', 'hiding', 'counties', 'effected', 'ji', 'certified', 'sustainable', 'ripping', 'abused', 'respected', 'equivalent', 'monetary', 'tuned', 'resident', 'models', 'photography', 'extension', 'indians', 'caution', 'subject', 'discretionary', 'aged', 'recipients', 'optimistic', 'rolling', 'lorry', 'piled', 'craft', 'malaria', 'covidindia', 'prioritize', 'copy', 'educate', 'seeks', 'newnormal', 'suspected', 'williams', 'heightened', 'context', 'collecting', 'holders', 'swear', 'beds', 'pos', 'hilarious', 'newyork', 'tipping', 'bond', 'logic', 'bacterial', 'throat', 'ho', 'hording', 'fr', 'btc', 'smith', 'quantity', 'guessing', 'cashing', 'chatting', 'arm', 'path', 'insider', 'addressing', 'loblaws', 'turmoil', 'surreal', 'cr', 'terrifying', 'lowes', 'appointment', 'processors', 'kentucky', 'infecting', 'blocked', 'targeting', 'vitamins', 'volumes', 'believes', 'career', 'score', 'refrain', 'strained', 'variety', 'meats', 'arvindkejriwal', 'proactive', 'courier', 'switched', 'lakh', 'impressed', 'matt', 'ethical', 'percentage', 'located', 'jordan', 'license', 'examines', 'kinds', 'mild', 'mindful', 'flood', 'vodka', 'somewhat', 'cryptocurrency', 'select', 'spots', 'display', 'migrant', 'shuts', 'walgreens', 'exclusively', 'containing', 'unknown', 'afloat', 'preventive', 'tries', 'churches', 'billionaires', 'parcel', 'scottmorrisonmp', 'weed', 'applications', 'olympics', 'immigrants', 'generous', 'bound', 'plummets', 'grain', 'broadband', 'stockpilers', 'factor', 'thinkwithgoogle', 'dire', 'interaction', 'drones', 'marked', 'theaters', 'collaboration', 'goal', 'pissed', 'sustainability', 'wks', 'pressures', 'gen', 'jason', 'wednesdaythoughts', 'cm', 'cfpb', 'govuk', 'robocalls', 'growers', 'confinement', 'ring', 'luckily', 'muslim', 'oflockdown', 'voted', 'chris', 'campus', 'overheard', 'apparel', 'theft', 'impending', 'port', 'dem', 'surveyed', 'december', 'mcdonald', 'solid', 'runwalofficial', 'trusted', 'representing', 'lmao', 'alerts', 'feedback', 'butchers', 'tight', 'messaging', 'nzherald', 'petrochemical', 'crush', 'kag', 'rightly', 'desperately', 'quarantines', 'offline', 'fargo', 'kn', 'marking', 'ample', 'confusion', 'cafe', 'taught', 'pacific', 'gaming', 'isnt', 'onion', 'intervention', 'pricegougers', 'fm', 'forum', 'saturdaymorning', 'resilience', 'drove', 'coronavirusau', 'seed', 'asdaserviceteam', 'holy', 'stranger', 'blows', 'retailnews', 'alot', 'relaxed', 'produces', 'player', 'startups', 'supports', 'massively', 'optimism', 'enjoying', 'fucked', 'exempt', 'ideal', 'fat', 'exception', 'serves', 'extraordinary', 'ufcw', 'repair', 'english', 'mohfw', 'exact', 'expands', 'karen', 'odisha', 'grew', 'contagious', 'ashley', 'railways', 'accc', 'disabilities', 'assess', 'scramble', 'inspired', 'artist', 'compliance', 'lifestyle', 'campaigns', 'sooner', 'kick', 'louis', 'pensioners', 'layer', 'nine', 'foreseeable', 'birds', 'rbi', 'differently', 'drawing', 'gulf', 'glut', 'adds', 'stats', 'winner', 'coronamemes', 'compiled', 'reliable', 'stands', 'fly', 'livestock', 'explaining', 'shelters', 'jacked', 'coronavirusinpakistan', 'chair', 'yrs', 'bracing', 'highlighted', 'lbs', 'absurd', 'stating', 'glass', 'thanking', 'grocer', 'frustrating', 'aimed', 'circle', 'mobility', 'entitled', 'roles', 'advising', 'positions', 'dwindling', 'heros', 'kimberly', 'band', 'nuts', 'carolina', 'coupons', 'aoc', 'fuckin', 'thursdaythoughts', 'thankfully', 'fracking', 'honor', 'queensland', 'countless', 'ounce', 'lonely', 'perfectly', 'relationship', 'populations', 'deeply', 'smoke', 'margins', 'dangers', 'separate', 'praying', 'safest', 'buck', 'horror', 'accelerated', 'evictions', 'fortunate', 'regulated', 'escape', 'annoying', 'hashtag', 'surprising', 'newly', 'sickness', 'losangeles', 'sanity', 'interactive', 'reminding', 'diary', 'hourly', 'hence', 'countdown', 'updating', 'deserved', 'kenyan', 'visitors', 'cops', 'install', 'stalls', 'til', 'ginger', 'salaries', 'villages', 'competing', 'argos', 'wk', 'nsw', 'cafes', 'announcements', 'recovering', 'sp', 'windows', 'feelings', 'sen', 'gowns', 'disturbing', 'nhsworkers', 'disney', 'aussie', 'pumping', 'alongside', 'zombies', 'admit', 'seasonal', 'yeast', 'initiatives', 'nightmare', 'solved', 'journey', 'handled', 'agent', 'va', 'mondaythoughts', 'preppers', 'relative', 'saferathome', 'supportlocal', 'registered', 'dis', 'expanding', 'huh', 'rethink', 'compassion', 'application', 'chancellor', 'washingtonpost', 'contribution', 'emarketer', 'alex', 'sanitized', 'incl', 'shale', 'colleague', 'swiss', 'adventure', 'portfolio', 'berlin', 'designs', 'exploitation', 'regulators', 'requested', 'proving', 'establishments', 'unlimited', 'doug', 'ball', 'netherlands', 'beaches', 'navigating', 'conspiracy', 'pockets', 'cmomaharashtra', 'rain', 'nhsheroes', 'dick', 'terror', 'mbs', 'determined', 'lifetime', 'halted', 'pants', 'waive', 'indiana', 'checkouts', 'database', 'travelling', 'deli', 'whiskey', 'hurricane', 'lick', 'randomly', 'gp', 'coronaalert', 'declare', 'woods', 'cybersecurity', 'desk', 'deeper', 'terroristic', 'nike', 'bernie', 'minimal', 'fulfill', 'downs', 'pathetic', 'whats', 'developments', 'unsafe', 'difficulty', 'fueled', 'declaration', 'influx', 'wic', 'egypt', 'keyworker', 'charts', 'tin', 'sensitive', 'younger', 'spx', 'october', 'regulation', 'pivot', 'freight', 'responds', 'approximately', 'gyms', 'pres', 'particles', 'addiction', 'served', 'ugly', 'lockdownextension', 'labels', 'prof', 'shake', 'cancelling', 'farmworkers', 'jeffbezos', 'haha', 'queens', 'rome', 'delivers', 'nail', 'scum', 'employ', 'cleanliness', 'suggesting', 'venmo', 'martin', 'ripple', 'outdoor', 'refuses', 'brain', 'assistants', 'engaging', 'spree', 'microsoft', 'fairly', 'dispute', 'upper', 'loyalty', 'adhere', 'transaction', 'deadline', 'bt', 'socialmedia', 'shaw', 'beast', 'screw', 'plain', 'moody', 'goals', 'engaged', 'bacon', 'loving', 'lawmakers', 'conduct', 'timing', 'poetry', 'violence', 'minimise', 'emergencies', 'loose', 'cigarettes', 'patel', 'khan', 'toxic', 'acceptable', 'appliances', 'boots', 'er', 'soul', 'facilitate', 'screening', 'acted', 'socal', 'method', 'efficient', 'prompts', 'pension', 'scarf', 'woolies', 'threaten', 'safetyfirst', 'wing', 'ppes', 'glove', 'stranded', 'golden', 'berniesanders', 'ottawa', 'fragile', 'handsanitizers', 'meetings', 'cooks', 'bandana', 'bbcbreakfast', 'pulled', 'fossil', 'yep', 'recruiting', 'tonnes', 'toilets', 'agri', 'foodservice', 'upends', 'joebiden', 'benchmark', 'bananas', 'licenses', 'gifts', 'whammy', 'device', 'ts', 'attached', 'shareholders', 'rolled', 'frontliners', 'networks', 'atta', 'confident', 'ethics', 'incident', 'accelerating', 'ing', 'observing', 'ordinary', 'clap', 'selected', 'thresholds', 'carefully', 'secret', 'mankind', 'chickens', 'mentalhealth', 'wheels', 'rot', 'investigating', 'function', 'replenish', 'classed', 'skilled', 'workplace', 'britons', 'mrx', 'sketch', 'grant', 'argue', 'senatemajldr', 'hackers', 'crossing', 'upside', 'sc', 'television', 'reopening', 'judge', 'errands', 'advisory', 'dependent', 'texans', 'interviewed', 'selection', 'telemedicine', 'alleviate', 'shaped', 'practising', 'sizes', 'isles', 'filter', 'consistent', 'vr', 'behalf', 'cbs', 'foodwaste', 'polite', 'realised', 'ct', 'settle', 'cre', 'forecasts', 'threatened', 'clinic', 'vegetarian', 'athletes', 'tremendous', 'maintenance', 'earned', 'admin', 'sheep', 'opt', 'dish', 'wa', 'ww', 'behavioral', 'anytime', 'pipeline', 'thousand', 'grants', 'despair', 'governance', 'therapy', 'prosecuted', 'ukraine', 'coop', 'ck', 'coronalockdown', 'chopped', 'slowed', 'hug', 'mounting', 'yelled', 'hosted', 'unacceptable', 'arrows', 'fordnation', 'typically', 'flooding', 'lord', 'segment', 'popping', 'drugstore', 'assistant', 'pot', 'dailymailuk', 'worldhealthday', 'afghanistan', 'aldiaustralia', 'improvement', 'onset', 'contestalert', 'sf', 'sunshine', 'genomics', 'transparency', 'reference', 'blowing', 'inner', 'ng', 'coronauk', 'workout', 'adhering', 'laptops', 'promoting', 'eaten', 'fan', 'pledge', 'seo', 'instructed', 'waiving', 'arseholes', 'hugely', 'ingredient', 'judging', 'harmful', 'fearful', 'sneezes', 'cleveland', 'feature', 'blaming', 'routes', 'fails', 'gang', 'haul', 'trials', 'processes', 'fishermen', 'robot', 'shoulder', 'slide', 'denied', 'ministers', 'dettol', 'tata', 'assessment', 'installed', 'leilani', 'discussed', 'butcher', 'tube', 'specially', 'predictions', 'staythefhome', 'aids', 'dumped', 'consumerbehavior', 'prospect', 'bins', 'vietnam', 'sheet', 'shaming', 'bout', 'alphabet', 'widely', 'aims', 'infographic', 'protein', 'sought', 'extent', 'evolve', 'crooks', 'import', 'inputs', 'entrepreneur', 'woke', 'warm', 'immigrant', 'arguing', 'expired', 'smallbusiness', 'tuna', 'looroll', 'reassure', 'pushes', 'panicbuy', 'unions', 'stem', 'decreases', 'herself', 'projected', 'gin', 'debit', 'generations', 'user', 'sobeys', 'leisure', 'bookings', 'nhsstaff', 'bradpaisley', 'estimate', 'guest', 'girls', 'records', 'dayslockdown', 'latter', 'nutritious', 'editor', 'ba', 'thin', 'ours', 'retailworkers', 'opposition', 'removing', 'pointing', 'loyal', 'enters', 'membership', 'spy', 'stash', 'relieve', 'applaud', 'irony', 'interactions', 'commit', 'onpoli', 'struggled', 'explode', 'nights', 'necessarily', 'alright', 'aggressive', 'artificial', 'elbow', 'owns', 'alarming', 'revolution', 'marketcrash', 'victoria', 'noticing', 'cunts', 'cbsnews', 'arab', 'fakenews', 'ukgoverment', 'pivoting', 'deficit', 'treats', 'fired', 'floridians', 'bleak', 'gaza', 'cheapest', 'wh', 'color', 'candy', 'uncertainties', 'plunges', 'proves', 'nhscovidheroes', 'proximity', 'blockchain', 'successfully', 'transmitted', 'explores', 'wallstreet', 'bastard', 'comfortable', 'alonetogether', 'cabinet', 'typical', 'disappear', 'centric', 'goodness', 'tables', 'negatively', 'intensifies', 'appalling', 'overcharging', 'wallet', 'alike', 'depth', 'sample', 'smartphone', 'rumours', 'apparent', 'disgrace', 'diamond', 'debate', 'piss', 'substitute', 'spoken', 'hanging', 'susceptible', 'obesity', 'privilege', 'cvs', 'downside', 'masked', 'shooting', 'coronavirusinsa', 'nc', 'processed', 'hint', 'roundup', 'parliament', 'forex', 'predicts', 'unite', 'comic', 'language', 'engagement', 'usps', 'approval', 'replace', 'rx', 'directed', 'trollies', 'med', 'steve', 'appealing', 'cmagovuk', 'curtail', 'tweeting', 'snack', 'addressed', 'spam', 'altered', 'owing', 'pelosi', 'tree', 'greece', 'mifi', 'milkman', 'assures', 'log', 'economically', 'pt', 'pulte', 'strip', 'breathe', 'penalties', 'nyse', 'shell', 'enormous', 'wi', 'century', 'bodies', 'goi', 'frightening', 'shipped', 'headquarters', 'fucks', 'latex', 'corps', 'vice', 'allocated', 'cereal', 'tragedy', 'majeure', 'quebec', 'earning', 'fool', 'occurred', 'nasty', 'named', 'nappies', 'hats', 'suburban', 'cooperation', 'tuesdaythoughts', 'merchandise', 'occupancy', 'reward', 'carried', 'breakingnews', 'labourers', 'damaged', 'supplements', 'chose', 'buildings', 'bits', 'notification', 'tackling', 'mac', 'obligations', 'grapples', 'ugh', 'arising', 'perceptions', 'smooth', 'mt', 'determine', 'rus', 'midwest', 'ranchers', 'wales', 'timeline', 'suck', 'cornwall', 'witness', 'condoms', 'politely', 'ce', 'document', 'printing', 'robots', 'mega', 'shirts', 'helpline', 'poorly', 'dating', 'hawaii', 'enterprise', 'qanon', 'joking', 'insulin', 'gauging', 'lawsuit', 'lee', 'shitty', 'cargo', 'performing', 'crew', 'pointed', 'del', 'wins', 'organized', 'automation', 'recruit', 'duties', 'tanks', 'christian', 'suspends', 'scores', 'claimed', 'stark', 'marijuana', 'skytv', 'november', 'smoking', 'facial', 'tomatoes', 'pune', 'washed', 'lamb', 'ebt', 'refugees', 'july', 'clip', 'cotton', 'gmail', 'pak', 'yellow', 'permission', 'wind', 'indonesia', 'confined', 'auction', 'mothers', 'attorneys', 'resume', 'cheat', 'vera', 'germ', 'moh', 'leverage', 'reaches', 'mount', 'realtor', 'kelly', 'logistical', 'pretending', 'combo', 'amitshah', 'arrangements', 'cruel', 'protocols', 'samples', 'coronaupdate', 'vendor', 'insist', 'euro', 'valued', 'raid', 'benefiting', 'trucking', 'alibaba', 'dreams', 'kevin', 'math', 'tourists', 'productive', 'funeral', 'undervalued', 'lineup', 'didnt', 'fold', 'bangladesh', 'defeat', 'protects', 'insanity', 'brussels', 'requiring', 'thoughtful', 'kirana', 'earthquake', 'utah', 'ratings', 'daniel', 'doc', 'elected', 'clapping', 'appearing', 'coverings', 'jewelry', 'cooked', 'moscow', 'waived', 'brace', 'kuwait', 'movements', 'flush', 'organised', 'pwc', 'prioritise', 'smiling', 'beings', 'container', 'vulnerability', 'unfairly', 'grabbing', 'duration', 'christ', 'italia', 'destroying', 'pulses', 'maharashtra', 'advanced', 'eve', 'staffs', 'laughed', 'diarrhea', 'cs', 'grabbed', 'signals', 'lasts', 'bent', 'ig', 'delaware', 'switzerland', 'hmm', 'gesture', 'historical', 'timely', 'licked', 'moral', 'flexibility', 'askebay', 'readers', 'reflects', 'ships', 'fortnight', 'shits', 'easyfundraising', 'sacrifices', 'dtc', 'directors', 'avail', 'wildlife', 'holds', 'unpaid', 'thoroughly', 'nycmayor', 'rentals', 'slammed', 'substantial', 'psychologist', 'reminded', 'logo', 'identity', 'rant', 'underway', 'concerning', 'kaduna', 'organisation', 'republican', 'makeup', 'meantime', 'garlic', 'museveni', 'weaker', 'pence', 'coronaviruspakistan', 'celebrating', 'lvmh', 'coronavirussa', 'twisted', 'factbox', 'dealt', 'respondents', 'paypal', 'scent', 'nonessential', 'clinical', 'canberra', 'presented', 'cinema', 'elite', 'journal', 'slower', 'payday', 'democrat', 'manufactured', 'expansion', 'harper', 'truce', 'cable', 'redistribution', 'plentiful', 'queued', 'detroit', 'beijing', 'pivots', 'foodsafety', 'iptv', 'redistribute', 'gcc', 'protectthenhs', 'informal', 'bump', 'icelandfoods', 'described', 'relationships', 'cma', 'taxpayers', 'tragic', 'retirement', 'millionaire', 'tablets', 'maine', 'pittsburgh', 'illustration', 'backed', 'thick', 'slip', 'attempts', 'arrange', 'exec', 'prevents', 'drama', 'printed', 'lane', 'subsequent', 'interim', 'imran', 'films', 'gained', 'ironic', 'fmi', 'squeeze', 'carrots', 'slows', 'incase', 'exporting', 'hidden', 'teen', 'remembered', 'contacts', 'isopropyl', 'counts', 'dankmemes', 'perspectives', 'hydro', 'teaching', 'rishisunak', 'posed', 'brian', 'lowers', 'pool', 'malicious', 'blind', 'distilling', 'dressed', 'rub', 'fred', 'quotes', 'japanese', 'bazaar', 'cater', 'abuja', 'theme', 'irish', 'foodsupply', 'bloomberg', 'radically', 'fixes', 'interests', 'hemp', 'glasgow', 'wal', 'rewards', 'tasks', 'abroad', 'furloughs', 'hometown', 'beg', 'damaging', 'commonsense', 'moron', 'shampoo', 'utterly', 'depending', 'decimated', 'eviction', 'forms', 'robbery', 'primarily', 'leg', 'lulu', 'carton', 'reeling', 'technologies', 'ray', 'representatives', 'concert', 'vans', 'polish', 'bankrupt', 'ktrtrs', 'accommodation', 'crews', 'gardening', 'stamp', 'af', 'rail', 'rand', 'fam', 'calgary', 'dilemma', 'flows', 'jumps', 'cookies', 'ha', 'shed', 'urgency', 'az', 'scientific', 'fatalities', 'exponentially', 'tn', 'searches', 'ate', 'presents', 'crisp', 'nascent', 'barren', 'depends', 'resort', 'linen', 'learnt', 'valid', 'complicated', 'library', 'patrons', 'controlling', 'stages', 'thesun', 'staggering', 'intervene', 'cmo', 'converted', 'waves', 'inventories', 'maddow', 'relation', 'filmed', 'ri', 'directive', 'sticking', 'plate', 'entrances', 'classic', 'pleasant', 'inspiration', 'nowadays', 'costing', 'specialist', 'respecting', 'opportunistic', 'spell', 'survivalist', 'ah', 'democratic', 'mailonline', 'brief', 'opinions', 'sentence', 'loaded', 'replied', 'newyorkcity', 'herald', 'sized', 'deserves', 'deputy', 'gst', 'dragged', 'nbcnews', 'yorkers', 'goggles', 'perishables', 'insightful', 'tumbled', 'grand', 'recycling', 'kidding', 'heartfelt', 'fulfillment', 'proposals', 'finminindia', 'bezos', 'ocd', 'nsitharaman', 'irvpaswan', 'guests', 'jerk', 'verizon', 'reporters', 'meets', 'coronavir', 'knees', 'marks', 'enact', 'receipt', 'trial', 'sex', 'naturally', 'fort', 'rounds', 'pneumonia', 'beverages', 'ripped', 'verify', 'loses', 'equipped', 'newspapers', 'premiums', 'harvesting', 'skill', 'waited', 'dig', 'technicians', 'ghost', 'abcnews', 'distillers', 'downloads', 'substantially', 'hamster', 'fares', 'beneficiaries', 'lung', 'normalcy', 'replacement', 'owe', 'transition', 'naira', 'technical', 'cousin', 'anticipation', 'quarantining', 'wishing', 'harvey', 'slap', 'deliveroo', 'nonprofits', 'unscrupulous', 'couriers', 'foodstuff', 'respectful', 'motion', 'dried', 'prayer', 'detail', 'spectrum', 'unity', 'praised', 'cyrilramaphosa', 'mate', 'entities', 'raided', 'socialdistancingnow', 'thehill', 'weighs', 'crack', 'brunt', 'signage', 'bro', 'tf', 'ab', 'mouths', 'born', 'chinesewuhanvirus', 'openings', 'coro', 'caregivers', 'indicating', 'punch', 'dna', 'assignments', 'panickbuyinguk', 'prioritizing', 'stated', 'binge', 'tfl', 'buybacks', 'arrival', 'portable', 'ids', 'ncov', 'rm', 'lanes', 'shore', 'subsides', 'trudeau', 'kate', 'exit', 'placing', 'avoided', 'observations', 'operator', 'accepted', 'pg', 'contained', 'governortomwolf', 'stars', 'apologise', 'ky', 'wednesdaywisdom', 'adopted', 'tennessee', 'iam', 'wrapped', 'represent', 'quicker', 'inspire', 'equal', 'junior', 'spat', 'preferred', 'automatic', 'gates', 'transformed', 'braved', 'readily', 'ish', 'witnessing', 'lpg', 'sparking', 'distant', 'chest', 'craziness', 'partial', 'abusing', 'conscious', 'mon', 'stakeholders', 'reactions', 'euros', 'overlooked', 'dealer', 'declares', 'pose', 'shs', 'crackdown', 'digest', 'antonio', 'litres', 'winners', 'courses', 'reshaping', 'bat', 'interviews', 'alcoholic', 'signal', 'blocks', 'compares', 'quarantineactivities', 'cardboard', 'eastersunday', 'anyways', 'gm', 'halal', 'sheer', 'apples', 'amazonuk', 'hoosiers', 'fractured', 'connections', 'screwed', 'furloughed', 'kleenex', 'fca', 'sensibly', 'coronavillains', 'reject', 'renting', 'negotiations', 'importers', 'coworkers', 'fintech', 'boomers', 'mystery', 'cartel', 'looming', 'flattening', 'flowing', 'purposely', 'shaping', 'wwii', 'teacher', 'violating', 'jim', 'pricks', 'bother', 'facetime', 'hubby', 'practise', 'storing', 'sigh', 'collapses', 'infrared', 'proved', 'mn', 'correctly', 'cow', 'appeared', 'ramen', 'sms', 'considers', 'dorset', 'msm', 'bengaluru', 'magnitude', 'ultra', 'restricting', 'celebration', 'musicians', 'sundaymorning', 'wed', 'profiteer', 'angel', 'visa', 'tonic', 'stricken', 'headline', 'alarm', 'legit', 'observation', 'surprisingly', 'ksa', 'miners', 'grandma', 'cupboard', 'bunker', 'investigated', 'revised', 'execs', 'mothersday', 'upward', 'lewis', 'sickening', 'myths', 'southern', 'courage', 'pe', 'designer', 'wuhancoronavirus', 'approaches', 'cue', 'positives', 'steam', 'cashless', 'queen', 'govts', 'pointless', 'skype', 'definition', 'shopkeeper', 'cracking', 'protest', 'routines', 'fijian', 'boyfriend', 'andy', 'cambridge', 'battered', 'planting', 'fedex', 'dine', 'lahore', 'symbol', 'figured', 'les', 'scenarios', 'universities', 'profile', 'theory', 'reassuring', 'powder', 'socialist', 'yell', 'filthy', 'barrier', 'tracked', 'tomato', 'dontpanic', 'hunker', 'susannareid', 'catches', 'srilanka', 'lka', 'brazil', 'pandemia', 'extensive', 'gazette', 'incindia', 'needless', 'involves', 'favor', 'mps', 'hungary', 'briefings', 'hyper', 'programme', 'keepyourdistance', 'outcomes', 'consumerprotection', 'classify', 'hearts', 'worlds', 'malware', 'wheel', 'waters', 'tougher', 'functional', 'celebrity', 'econ', 'anticipated', 'arriving', 'humanitarian', 'aviation', 'realistic', 'publication', 'fridges', 'sum', 'strikes', 'uh', 'verbally', 'creativity', 'apologize', 'begging', 'length', 'commentary', 'borrowers', 'reviews', 'webpage', 'acknowledge', 'picknpay', 'communicate', 'structure', 'outlet', 'superheroes', 'weapon', 'terrorism', 'prevailing', 'equally', 'postmen', 'regard', 'lemon', 'honey', 'narrative', 'rep', 'pads', 'consequence', 'grace', 'ram', 'eh', 'ltd', 'sneezed', 'airports', 'prescriptions', 'unusual', 'fitting', 'progress', 'moaning', 'essays', 'mcdonalds', 'tyson', 'bingo', 'crushed', 'generated', 'instance', 'primark', 'stance', 'spaghetti', 'compensate', 'propose', 'promotion', 'receives', 'catastrophic', 'respectively', 'emts', 'toiletpapershortage', 'prospects', 'automatically', 'internal', 'prepares', 'retired', 'candidate', 'presence', 'threw', 'tumbling', 'dystopian', 'grossly', 'fridayfeeling', 'liverpool', 'soybean', 'peas', 'relate', 'species', 'illnesses', 'kidney', 'cushion', 'californialockdown', 'brescia', 'philly', 'stream', 'partnered', 'aspects', 'cakes', 'kicks', 'unreal', 'consumed', 'kitchens', 'bragging', 'raiding', 'soared', 'encourages', 'purposes', 'protocol', 'phoenix', 'pending', 'astronomical', 'genuine', 'imf', 'mainstream', 'mills', 'participating', 'bite', 'worn', 'laughter', 'intensive', 'collections', 'lacking', 'incompetent', 'core', 'believed', 'communist', 'shrinking', 'wereinthistogether', 'suffered', 'wood', 'eagle', 'weapons', 'youre', 'birth', 'shameless', 'privileged', 'sephora', 'coronavirussouthafrica', 'whereas', 'bomb', 'essentially', 'coronavirusnz', 'detailed', 'npr', 'sustain', 'doomsday', 'amzn', 'carrefour', 'hon', 'murphy', 'bestbuy', 'distanced', 'symptom', 'bangkok', 'mandated', 'ect', 'dedicate', 'howard', 'propaganda', 'noted', 'counting', 'spin', 'tour', 'footing', 'addresses', 'influencing', 'latin', 'coronavirusinindia', 'homebound', 'fatal', 'premises', 'broad', 'sprayed', 'fourth', 'clarify', 'laptop', 'lights', 'stimulate', 'afp', 'korean', 'stephen', 'advertise', 'mrp', 'ni', 'gender', 'checklist', 'heck', 'artificially', 'phil', 'pin', 'deferred', 'justsaying', 'structural', 'religion', 'holdings', 'spiking', 'tory', 'laboratory', 'robert', 'shopritestores', 'fitness', 'pairs', 'camp', 'belt', 'weddings', 'stake', 'mary', 'reform', 'lifting', 'tobacco', 'sweeping', 'tampa', 'unitedstates', 'versus', 'homeschooling', 'frame', 'legitimate', 'ii', 'appropriately', 'railway', 'disinfection', 'beers', 'magazine', 'supplychains', 'packing', 'clarity', 'reliant', 'bagging', 'lazy', 'shine', 'sheltering', 'sap', 'alleged', 'spiral', 'inability', 'avenue', 'ventured', 'dollartree', 'gary', 'belgium', 'batch', 'merch', 'pattern', 'quarterly', 'sends', 'unchanged', 'honour', 'uninsured', 'coronainpakistan', 'indiafightscoronavirus', 'wankers', 'li', 'survivor', 'outlines', 'securing', 'evolution', 'lake', 'sanitization', 'ave', 'clowns', 'answering', 'venturing', 'nebraska', 'absence', 'danielandrewsmp', 'solely', 'convinced', 'weakened', 'sciences', 'ubereats', 'lyft', 'attacking', 'poses', 'exploring', 'nicely', 'rationed', 'believing', 'contrast', 'biscuits', 'refer', 'falsely', 'boycotthul', 'flower', 'inadequate', 'springs', 'consuming', 'digit', 'reader', 'staffing', 'reflected', 'su', 'advises', 'janatacurfew', 'bbcbreaking', 'curfews', 'outfit', 'inconsiderate', 'atlantic', 'wishes', 'insensitive', 'pilot', 'idk', 'transfers', 'killer', 'donors', 'entertain', 'cont', 'tire', 'mkt', 'depleted', 'adversity', 'elders', 'contingency', 'conservatives', 'papertowels', 'lbc', 'immense', 'intend', 'mentality', 'whatsoever', 'cp', 'cried', 'artisanal', 'bcpoli', 'nys', 'mbuhari', 'micro', 'yoga', 'reckon', 'twats', 'iranian', 'gram', 'plane', 'coincidence', 'frm', 'penny', 'tear', 'chef', 'stricter', 'trapped', 'itvnews', 'transformation', 'approx', 'asses', 'wire', 'bats', 'thomas', 'wary', 'dang', 'woes', 'completed', 'complications', 'jennifer', 'gougers', 'ransacked', 'indicative', 'obey', 'discussions', 'charitable', 'inspiring', 'unsolicited', 'rushed', 'colours', 'august', 'utmost', 'nm', 'features', 'warriors', 'paused', 'william', 'gal', 'mi', 'tensions', 'imrankhanpti', 'firemen', 'transunion', 'kansas', 'wonders', 'arent', 'insufficient', 'setup', 'audience', 'pharmaceuticals', 'exacerbated', 'depressing', 'buddy', 'suspicious', 'promising', 'floors', 'americas', 'fools', 'enabling', 'burn', 'subsidy', 'neighborhoods', 'richest', 'frequent', 'drag', 'sprays', 'assuming', 'preserve', 'secondary', 'karnataka', 'busch', 'maize', 'sewing', 'indicates', 'easing', 'gaps', 'medics', 'relatives', 'emerged', 'hmmm', 'creators', 'inviting', 'poorer', 'questionable', 'acquired', 'standstill', 'unskilled', 'fijinews', 'adopt', 'oilandgas', 'halloween', 'dankmeme', 'poorest', 'seriousness', 'mistakes', 'savers', 'advocacy', 'racing', 'faqs', 'civilization', 'hd', 'tied', 'sec', 'outdoors', 'paris', 'registration', 'philippines', 'curbs', 'battles', 'tattoo', 'lawsuits', 'ethiopia', 'purposefully', 'complimentary', 'stayathomeandstaysafe', 'objects', 'newsom', 'iga', 'clues', 'helpeachother', 'ausbiz', 'kaikohe', 'studying', 'sessions', 'dispensers', 'manitoba', 'momentum', 'italians', 'obama', 'layers', 'wfp', 'dividends', 'josh', 'accessories', 'hydcitypolice', 'moreover', 'browse', 'furlough', 'advocate', 'drew', 'nclc', 'homeowners', 'unfolding', 'flyfrontier', 'hospitalized', 'grapple', 'attending', 'simon', 'ssa', 'recap', 'iraqi', 'doom', 'responder', 'installing', 'accessing', 'improved', 'mtn', 'quoted', 'di', 'functioning', 'sanitising', 'atms', 'fare', 'reductions', 'sport', 'presidential', 'seat', 'browsing', 'coronaviruscanada', 'cooper', 'overpricing', 'essentialworker', 'circuit', 'wisdom', 'mutual', 'dash', 'robbing', 'overdrive', 'sourcing', 'gauge', 'gears', 'volunteering', 'superstore', 'fdic', 'unused', 'jet', 'troops', 'specialists', 'waking', 'scored', 'saturdaythoughts', 'allowance', 'stall', 'bullish', 'bird', 'scottish', 'portion', 'realizing', 'buffer', 'afterwards', 'opposed', 'lupus', 'oils', 'gradually', 'theirs', 'lobby', 'demonstrates', 'cod', 'rout', 'crashes', 'belief', 'unfortunate', 'bbl', 'residence', 'xmas', 'reserving', 'gouge', 'uhuru', 'clown', 'alliance', 'recognised', 'reschedule', 'linger', 'spiked', 'broader', 'mfs', 'organize', 'equities', 'actress', 'graphic', 'wereallinthistogether', 'congratulations', 'ripoff', 'keepers', 'jamie', 'offs', 'nl', 'directing', 'touches', 'define', 'mexican', 'mortality', 'clears', 'petrolprice', 'cancels', 'webinars', 'thinkofothers', 'edmonton', 'billionaire', 'stringent', 'chronic', 'chinaliedpeopledied', 'consumerconfidence', 'fooddelivery', 'ff', 'poised', 'marketer', 'memory', 'mustard', 'piles', 'togetherathome', 'directives', 'meijer', 'cocaine', 'morgan', 'currencies', 'spark', 'honorable', 'likelihood', 'alternate', 'relies', 'gilead', 'bunny', 'rubbish', 'chip', 'flyers', 'distances', 'decides', 'tale', 'limitations', 'chase', 'circulating', 'terminal', 'footage', 'column', 'arrives', 'freak', 'upto', 'embrace', 'boosts', 'conducting', 'beard', 'importing', 'odds', 'uncharted', 'wth', 'chinesecoronavirus', 'thewalkingdead', 'diego', 'vary', 'inbox', 'catering', 'wicked', 'eleven', 'bloke', 'striking', 'exams', 'escalate', 'ja', 'gld', 'denial', 'bumping', 'climb', 'married', 'pity', 'intensified', 'safeguards', 'dal', 'etiquette', 'ugandans', 'servants', 'practiced', 'brewing', 'bridge', 'salman', 'provinces', 'wwe', 'recommending', 'consultant', 'bangalore', 'newzealand', 'android', 'regulating', 'rbc', 'clueless', 'hobby', 'lobbying', 'accidental', 'warehousing', 'toss', 'hmu', 'principal', 'strengthen', 'allegations', 'republic', 'quietly', 'livelihood', 'impressive', 'venues', 'drought', 'partnering', 'undocumented', 'cop', 'horizon', 'senatorloeffler', 'epa', 'automated', 'badge', 'journeys', 'continuity', 'victory', 'applicable', 'jane', 'mg', 'generate', 'competitors', 'windsor', 'coronapandemic', 'arkansas', 'chevron', 'dia', 'indialockdown', 'simulation', 'mena', 'overview', 'clapforcarers', 'wearamask', 'analyzed', 'coronavirustruth', 'violated', 'contractions', 'denying', 'clapforkeyworkers', 'flaws', 'adoting', 'ayurved', 'bailing', 'largo', 'tariff', 'christianity', 'smithfield', 'km', 'winery', 'licensed', 'yorkshire', 'exposes', 'reps', 'glimpse', 'markings', 'tees', 'meyer', 'jacindaardern', 'excessively', 'jeffreestar', 'pivoted', 'subscriptions', 'initially', 'orleans', 'shielding', 'zambia', 'sheffield', 'stayathomeorder', 'docs', 'miracle', 'hotspots', 'hyd', 'disastrous', 'adoption', 'alabama', 'engineer', 'kerala', 'calculated', 'entity', 'bull', 'weighing', 'busier', 'citing', 'sponsored', 'confronted', 'branding', 'selfless', 'beaten', 'slides', 'scumbags', 'gaining', 'lisa', 'respite', 'prince', 'backbone', 'predicting', 'acceptance', 'lawyers', 'dynamic', 'imminent', 'louisiana', 'split', 'supermarketsweep', 'dems', 'seats', 'pledged', 'slim', 'bids', 'loot', 'russians', 'delaying', 'pleading', 'goodbye', 'label', 'syria', 'meaningful', 'imo', 'brutal', 'aluminium', 'trumps', 'aaa', 'burr', 'devil', 'hassle', 'crimes', 'slams', 'nbc', 'moronic', 'vuitton', 'borrow', 'newest', 'pages', 'bekindtoeachother', 'coronaindia', 'bye', 'deployed', 'pti', 'portugal', 'harry', 'seized', 'swan', 'phe', 'peanut', 'sterling', 'demanded', 'troubled', 'lease', 'wreaking', 'daddy', 'stressing', 'bum', 'directions', 'riyadh', 'applying', 'lime', 'finnish', 'mitigation', 'planners', 'resale', 'adjusts', 'freely', 'legally', 'kicking', 'homedepot', 'audio', 'imperative', 'familiar', 'mama', 'biden', 'borrowing', 'rallying', 'gr', 'rocket', 'incentive', 'disappeared', 'laying', 'monopoly', 'yelp', 'horse', 'researching', 'bizarre', 'accountability', 'doubles', 'securities', 'male', 'ages', 'minus', 'celebrated', 'mayhem', 'federation', 'emissions', 'jam', 'arse', 'socks', 'pacp', 'appointments', 'fi', 'stimuluspackage', 'relations', 'accommodate', 'rinse', 'cage', 'mistake', 'capitalize', 'bites', 'sandwich', 'apocalyptic', 'rumors', 'grandmother', 'proportion', 'screaming', 'goldman', 'exercising', 'title', 'vat', 'sacrificing', 'chaotic', 'livelihoods', 'appalled', 'ted', 'manner', 'transparent', 'loves', 'dedication', 'plays', 'cigarette', 'fires', 'rides', 'informative', 'indication', 'declaring', 'pig', 'columbia', 'prompting', 'tops', 'weigh', 'agenda', 'reselling', 'discriminate', 'deploy', 'nov', 'covidontario', 'coronavirusitaly', 'brewery', 'pills', 'copd', 'practitioners', 'utilize', 'aiming', 'floating', 'fearing', 'fried', 'lawyer', 'pigs', 'yeg', 'abandoned', 'recording', 'excise', 'mountain', 'horrendous', 'northeast', 'stockmarketcrash', 'clever', 'exporter', 'animalcrossing', 'bravo', 'computers', 'veggie', 'nt', 'stretch', 'accordingly', 'disappointing', 'goodnews', 'orlando', 'encountered', 'bean', 'theatre', 'annoyed', 'profound', 'inevitably', 'riot', 'shoe', 'prohibits', 'hordes', 'nordstrom', 'swing', 'travelers', 'taped', 'bouncing', 'floods', 'powered', 'flyer', 'medically', 'uni', 'mayorofla', 'birmingham', 'beating', 'religious', 'awhile', 'furious', 'entertained', 'baggers', 'flex', 'chapter', 'riders', 'buttons', 'porters', 'dontbeaspreader', 'pint', 'yomequedoencasa', 'markers', 'climatechange', 'salad', 'dave', 'authorized', 'onions', 'licences', 'mandate', 'lebanon', 'sane', 'litter', 'rack', 'sandwiches', 'branches', 'freezing', 'electronic', 'hack', 'evident', 'fragility', 'beloved', 'monster', 'develops', 'soda', 'compound', 'tie', 'staring', 'sos', 'politician', 'gels', 'settled', 'voters', 'credits', 'inconvenience', 'chinaliedandpeopledied', 'applause', 'bogroll', 'chewy', 'kumar', 'obtain', 'unleaded', 'gathered', 'outcome', 'disinformation', 'telanganacmo', 'lemons', 'exporters', 'hardworking', 'deflation', 'publishers', 'resolve', 'turbulent', 'sisters', 'discrimination', 'advent', 'tanking', 'tribute', 'hook', 'deny', 'balcony', 'touted', 'capping', 'fijians', 'turnover', 'mercy', 'cleans', 'dot', 'footfall', 'spokesperson', 'cycling', 'featured', 'introduces', 'lined', 'grim', 'popularity', 'nan', 'spaced', 'blogs', 'polling', 'mechanism', 'rainbow', 'wireless', 'bowl', 'painful', 'wwd', 'lauren', 'ccpvirus', 'writer', 'ncdcgov', 'ev', 'bil', 'posing', 'prolonged', 'avg', 'bold', 'margin', 'considerations', 'peaks', 'influenza', 'liberals', 'bootsuk', 'staffed', 'altogether', 'gps', 'earrings', 'trajectory', 'regret', 'defining', 'thai', 'wv', 'seating', 'denver', 'scientist', 'kent', 'supporters', 'unstable', 'positivity', 'hustle', 'jared', 'intelligent', 'tone', 'prioritized', 'dual', 'checkers', 'nick', 'amend', 'brampton', 'colors', 'negotiate', 'dowjones', 'pretend', 'economiccrisis', 'il', 'cv', 'discard', 'jantacurfew', 'promises', 'qatarairways', 'sue', 'minority', 'residentevil', 'invisible', 'pharmacist', 'learnings', 'harms', 'decreasing', 'dignity', 'saturated', 'trumppressconference', 'enhance', 'engineers', 'sunglasses', 'gut', 'principles', 'convert', 'journalist', 'scan', 'toiletpaperwars', 'ridiculously', 'cheer', 'saliva', 'liter', 'edibles', 'patanjali', 'techs', 'thieves', 'snake', 'highway', 'coronastopkarona', 'exploited', 'teamed', 'fleeing', 'salvation', 'um', 'brains', 'pilling', 'israeli', 'invited', 'ore', 'bilbrough', 'favour', 'punjab', 'dye', 'dublin', 'worsening', 'swiftly', 'coronavirusinsouthafrica', 'ports', 'insecure', 'reforms', 'loud', 'sued', 'medicaid', 'sincerely', 'nets', 'components', 'amazonfresh', 'collectively', 'practically', 'nfl', 'retweeet', 'uncle', 'recognition', 'scrap', 'saveonfoods', 'allies', 'shortly', 'mil', 'domino', 'malaysialockdown', 'inundated', 'reflection', 'appetite', 'mums', 'fisher', 'profitability', 'speculation', 'tens', 'greeter', 'trap', 'blown', 'distinct', 'congrats', 'brink', 'leaning', 'licence', 'oriented', 'ss', 'ht', 'workplaces', 'vision', 'flooded', 'upping', 'touchscreens', 'drfauci', 'void', 'chosen', 'globaldata', 'prescribed', 'loeffler', 'exceptions', 'susan', 'mcconnell', 'corporates', 'spotlight', 'federalreserve', 'delighted', 'branded', 'washes', 'upstream', 'teenager', 'codvid', 'hugs', 'togetherapart', 'comedian', 'blessing', 'withstand', 'sindh', 'yields', 'shelteringinplace', 'focuses', 'par', 'ink', 'disinfected', 'shoponline', 'turkish', 'titles', 'hy', 'eurozone', 'anna', 'treasury', 'instantly', 'wines', 'bengal', 'jamaica', 'demographic', 'coke', 'mentally', 'wrap', 'suppress', 'discovering', 'rings', 'defer', 'fluid', 'ensures', 'singh', 'shouted', 'abundant', 'vile', 'daughters', 'outs', 'ffp', 'pressuring', 'tours', 'river', 'explosion', 'diagnostic', 'kenney', 'tempted', 'tricks', 'surrey', 'enables', 'martinslewis', 'coordinated', 'parade', 'rv', 'sixfeetapart', 'sri', 'beats', 'ignite', 'shining', 'seemingly', 'hesitate', 'montana', 'localbusiness', 'ang', 'bidets', 'staypositive', 'coopuk', 'newjersey', 'warming', 'poo', 'moon', 'surgery', 'settings', 'versions', 'occurs', 'christchurch', 'immigration', 'beside', 'manhattan', 'dodging', 'ecb', 'wandering', 'dominos', 'firing', 'abundance', 'reduceinternetprices', 'closest', 'dodge', 'sheeple', 'uniform', 'memories', 'staggered', 'wondered', 'tier', 'communicating', 'butt', 'forgetting', 'holes', 'oxygen', 'sides', 'animation', 'adobe', 'annotated', 'savvy', 'hazmat', 'sweden', 'esg', 'humour', 'assessing', 'liberal', 'adequately', 'acute', 'fantasy', 'worsen', 'hammered', 'poisoning', 'holder', 'emi', 'enterprises', 'mint', 'simultaneously', 'songs', 'homedelivery', 'ranks', 'sunbathing', 'detection', 'municipal', 'giantfood', 'ceiling', 'shrink', 'pensions', 'fri', 'restoring', 'viable', 'journalism', 'dmv', 'costly', 'dynata', 'everytime', 'financing', 'coworker', 'designation', 'yield', 'scrambles', 'skyrockets', 'marketwatch', 'stew', 'fundamentally', 'fortunately', 'algeria', 'jaketapper', 'nationally', 'consumerrights', 'camps', 'detention', 'violations', 'shaking', 'kim', 'disproportionately', 'consistently', 'venezuelan', 'yelling', 'swell', 'dirt', 'cluster', 'braving', 'notices', 'subscribe', 'adelaide', 'files', 'kiss', 'banana', 'toddler', 'reassured', 'crushes', 'tvs', 'lookout', 'labreports', 'hum', 'bibliographies', 'ramifications', 'slipped', 'occurring', 'shots', 'aliens', 'bets', 'capable', 'luzon', 'diagnosis', 'riding', 'keepcalm', 'pp', 'ty', 'kashmir', 'auctions', 'hypocrisy', 'ilorin', 'travellers', 'quarentine', 'mates', 'formed', 'twin', 'pens', 'sm', 'insisted', 'chamber', 'debbie', 'makro', 'fiction', 'faq', 'filing', 'maintained', 'immoral', 'foreclosures', 'expenditure', 'loop', 'scammed', 'thx', 'disrupting', 'doubts', 'emphasis', 'coronalockdownuk', 'elevator', 'anxieties', 'hundred', 'pitch', 'dat', 'wen', 'canonforcommunity', 'ranging', 'caribbean', 'magic', 'adam', 'pulp', 'overrun', 'triggers', 'nessel', 'andme', 'assisting', 'charles', 'perform', 'rating', 'nah', 'blamed', 'profitable', 'dominance', 'coronovirus', 'vacuum', 'toiletrollchallenge', 'separation', 'slips', 'sovereign', 'generic', 'int', 'convince', 'tension', 'schoolclosuresuk', 'evolves', 'prioritising', 'outstanding', 'releasing', 'usatoday', 'murder', 'productivity', 'dms', 'cfo', 'moms', 'caresact', 'recovers', 'radius', 'participants', 'conservative', 'clinics', 'predatory', 'encounter', 'influencers', 'billing', 'ubi', 'listened', 'skimming', 'asians', 'tuckercarlson', 'recognizing', 'luxembourg', 'grandparent', 'retailing', 'greeted', 'tray', 'recruitment', 'peterpsquare', 'tesla', 'islands', 'forrester', 'plates', 'commercials', 'disposal', 'charlotte', 'virologist', 'irgc', 'welp', 'discipline', 'ive', 'madrid', 'aunt', 'realtime', 'massgovernor', 'reset', 'rocketing', 'crunch', 'lockdownaustralia', 'safeguarding', 'underground', 'excuses', 'harris', 'threshold', 'cpi', 'prompt', 'essay', 'overpriced', 'oversight', 'forth', 'daunt', 'cup', 'aircanada', 'coimbatore', 'pour', 'achieved', 'wwg', 'spglobalplatts', 'iron', 'mentions', 'stares', 'weekends', 'critically', 'league', 'mla', 'deborah', 'mypov', 'refrigeration', 'museums', 'sanders', 'outta', 'ne', 'emailed', 'diamonds', 'tall', 'cab', 'degrees', 'diapers', 'fancy', 'consecutive', 'fundamentals', 'recessions', 'delta', 'stole', 'govinslee', 'du', 'bopinion', 'ns', 'highriskcovid', 'wmt', 'restore', 'lands', 'township', 'sears', 'anonymous', 'aaron', 'matthew', 'replaced', 'sporting', 'hhs', 'lo', 'royalmail', 'compulsory', 'infused', 'fg', 'smells', 'indicate', 'treasure', 'dotherightthing', 'joseph', 'basement', 'soo', 'desire', 'inter', 'weston', 'landlord', 'airbnb', 'cooperate', 'happily', 'aspect', 'waterstones', 'noise', 'tonne', 'fuckers', 'gmt', 'bearish', 'silent', 'ei', 'tw', 'trumppandemic', 'amen', 'selfishpeople', 'shaken', 'replenished', 'grave', 'taskforce', 'pulls', 'heat', 'guinea', 'leeds', 'ebola', 'repurposed', 'virtue', 'ahmed', 'digitally', 'fate', 'stayhomesavelifes', 'spice', 'baseball', 'easterweekend', 'ios', 'yyc', 'tracks', 'tighter', 'karachi', 'squares', 'measured', 'shanghai', 'stpatricksday', 'portions', 'argues', 'trillions', 'peril', 'telangana', 'nairobi', 'coronavirusinnigeria', 'ditch', 'threeuk', 'jimmy', 'malawi', 'moisturizing', 'garylineker', 'stone', 'grass', 'bcoz', 'yaffebellany', 'scarsdale', 'wakeup', 'craze', 'sentiments', 'essentialservices', 'tunnel', 'coalition', 'migrants', 'classy', 'exceptional', 'turnip', 'lens', 'bharat', 'amy', 'panick', 'makeshift', 'stickers', 'salon', 'deferrals', 'wena', 'hannaford', 'disregard', 'coordination', 'cocoa', 'approaching', 'mylan', 'lobster', 'lacs', 'antitrust', 'cor', 'arrests', 'evicted', 'spgmarketintel', 'trumpmeltdown', 'radical', 'jidesanwoolu', 'gouged', 'abide', 'intense', 'sanfrancisco', 'sto', 'imposter', 'incarcerated', 'drain', 'wisely', 'ac', 'shutter', 'workouts', 'feared', 'elonmusk', 'lockdownsouthafrica', 'antiseptic', 'anheuser', 'palm', 'pleads', 'theage', 'handful', 'gofundme', 'coronavirusinkenya', 'hints', 'amazonsmile', 'allen', 'integrity', 'experiment', 'sorting', 'mondaymotivaton', 'conquer', 'skincare', 'olds', 'renters', 'bathrooms', 'traveled', 'speakers', 'vee', 'filters', 'smartphones', 'ruin', 'overreacting', 'mtnng', 'error', 'ratio', 'fought', 'tortillas', 'bitches', 'steak', 'frustration', 'violation', 'deciding', 'alan', 'disappointment', 'cargill', 'certificates', 'fbcnews', 'betting', 'ye', 'govrondesantis', 'improving', 'bake', 'salem', 'unpredictable', 'memorial', 'dakota', 'defraud', 'momlife', 'onlinemarketing', 'sem', 'insure', 'nationals', 'relaxing', 'stresses', 'checkerssa', 'advertisers', 'miserable', 'austerity', 'extras', 'stopandshop', 'hunter', 'nancy', 'rocked', 'clippers', 'coronapandemie', 'mondaymood', 'tradition', 'warren', 'prognosis', 'disrupts', 'governorva', 'fairprice', 'faring', 'provincial', 'haircut', 'ron', 'ear', 'panama', 'efficiently', 'savages', 'heights', 'advisors', 'healthcareheroes', 'ndtv', 'expectation', 'bankruptcies', 'scanning', 'spices', 'accident', 'krg', 'curbed', 'weakness', 'receipts', 'isolationlife', 'doorsteps', 'thier', 'rig', 'smes', 'sponsor', 'oblivious', 'arthritis', 'simpler', 'libya', 'bopis', 'sanitise', 'eased', 'nearing', 'milan', 'pessimism', 'raging', 'bazar', 'debenhams', 'mondaymorning', 'ebook', 'realjameswoods', 'speaker', 'handmade', 'dth', 'supporter', 'inches', 'coronatime', 'mol', 'santa', 'pictured', 'africans', 'acc', 'weareallinthistogether', 'pickers', 'retreats', 'activated', 'yay', 'scratch', 'assam', 'expertise', 'confront', 'speedy', 'refrigerators', 'affluent', 'statements', 'ryan', 'guelph', 'author', 'taylor', 'boxing', 'discourage', 'loaves', 'para', 'comeback', 'festival', 'semiconductor', 'madison', 'paranoia', 'teamwork', 'bankofamerica', 'ta', 'autoimmune', 'meltdown', 'understands', 'scanner', 'graduate', 'res', 'incidents', 'agrees', 'ics', 'tr', 'attract', 'soooo', 'pickups', 'pill', 'frustrated', 'abusive', 'pleasantly', 'nevada', 'casino', 'bargains', 'pipe', 'rwandans', 'catastrophe', 'ipa', 'varying', 'operates', 'seekers', 'advisor', 'vultures', 'cinemas', 'prosecute', 'resell', 'breeding', 'originally', 'problematic', 'browns', 'mere', 'bucks', 'lone', 'niece', 'coviduk', 'shoppingonline', 'txlege', 'grips', 'wolf', 'monitors', 'thursdaymotivation', 'govsisolak', 'bristol', 'dependence', 'login', 'shouting', 'andrex', 'healthier', 'sn', 'resign', 'aramco', 'chick', 'piano', 'lockdownlondon', 'attractive', 'amazed', 'childhood', 'medal', 'adviser', 'darkness', 'cagovernor', 'throws', 'havent', 'hugging', 'bolster', 'choosing', 'verge', 'hay', 'renewables', 'sorted', 'uneducated', 'algorithms', 'ctvnews', 'soldout', 'nhsengland', 'pan', 'unforeseen', 'elder', 'taxpayer', 'maps', 'solar', 'cracks', 'finishing', 'pun', 'barcelona', 'wembley', 'stack', 'graph', 'spraying', 'careers', 'belfast', 'fortune', 'diligently', 'goddamn', 'nicer', 'bakeries', 'pcs', 'donaldtrump', 'heartless', 'cocktail', 'diagnose', 'marketplaces', 'idle', 'represents', 'chasing', 'contraction', 'ralph', 'handsanitiser', 'amazonin', 'worsens', 'ind', 'ought', 'unethical', 'nifty', 'stuffed', 'tescos', 'barometer', 'quarantinecats', 'swedish', 'passenger', 'orgs', 'sardines', 'communism', 'cheeks', 'lessen', 'der', 'remake', 'djia', 'cautions', 'invented', 'imagined', 'hired', 'sing', 'paswan', 'engineering', 'rampant', 'conversations', 'jeremycorbyn', 'targets', 'stat', 'cries', 'altering', 'webcast', 'jhootspharmacy', 'durables', 'desert', 'droves', 'metrics', 'bakers', 'coronavirusnigeria', 'aggressively', 'gloom', 'fuckwits', 'cl', 'instruction', 'gta', 'upheaval', 'vastly', 'fbi', 'taiwan', 'girlfriend', 'apartments', 'balls', 'cornavirusoutbreak', 'regain', 'coombs', 'http', 'antibodies', 'endangering', 'laboratories', 'emotionally', 'savelives', 'describes', 'medtwitter', 'shortfall', 'attempted', 'skyhelpteam', 'biotech', 'philippine', 'cabin', 'selfemployed', 'understandable', 'instore', 'graham', 'jackson', 'installment', 'saint', 'fluctuations', 'sanctuary', 'menards', 'henry', 'goodwill', 'respirator', 'arguments', 'considerably', 'dare', 'porch', 'burning', 'qe', 'moci', 'seasons', 'inflicted', 'govlarryhogan', 'cape', 'freaked', 'sunny', 'protectyourself', 'ccpa', 'submitted', 'rarely', 'giveaway', 'borough', 'hype', 'rewe', 'alaska', 'conferences', 'ghanaian', 'ch', 'screamed', 'tel', 'lethal', 'voteblue', 'tide', 'realtors', 'angels', 'manners', 'hyvee', 'morale', 'staythefuckhome', 'richmond', 'connectivity', 'distributor', 'shud', 'gross', 'actor', 'tripling', 'dj', 'debts', 'aud', 'substitutes', 'suffers', 'joints', 'yard', 'fifth', 'forty', 'heroic', 'dprinting', 'defective', 'obsession', 'reopened', 'prepayment', 'ears', 'cu', 'legend', 'salons', 'manufactures', 'blank', 'remedies', 'docks', 'suitable', 'constraints', 'establish', 'newworldorder', 'commits', 'behaved', 'schooling', 'foresee', 'frankly', 'expense', 'concerts', 'abiding', 'trevornoah', 'thriving', 'satire', 'dentists', 'customerservice', 'frantic', 'cloths', 'homework', 'lighting', 'mayoroflondon', 'hacks', 'unwanted', 'scrutiny', 'irrational', 'emailing', 'tower', 'asx', 'invoke', 'jumia', 'visual', 'nitrile', 'ut', 'stateofemergency', 'diabetic', 'scammer', 'campbell', 'animalcrossingnewhorizons', 'cells', 'halting', 'cruises', 'todo', 'saver', 'dec', 'sikh', 'vladimir', 'pleas', 'argentina', 'swift', 'ctsi', 'doesnt', 'austria', 'basketball', 'pple', 'spencer', 'tpshortage', 'courts', 'ammunition', 'memo', 'boring', 'tanzania', 'vid', 'authors', 'foodshortage', 'scamalert', 'franchise', 'dime', 'payers', 'aldiusa', 'settles', 'blitz', 'gc', 'bob', 'lifesaving', 'swimming', 'flushing', 'demo', 'ulta', 'traderjoes', 'worsened', 'covidbc', 'renewable', 'geared', 'assault', 'cking', 'preventative', 'neck', 'sausage', 'accuracy', 'nintendo', 'tend', 'recognise', 'orderly', 'terribly', 'jimcramer', 'spends', 'argument', 'slice', 'heist', 'advisories', 'recognized', 'earnest', 'luke', 'auckland', 'vibe', 'marketresearch', 'environments', 'vaccinations', 'faculty', 'ko', 'govmikedewine', 'cunt', 'yummy', 'veteran', 'prisons', 'ani', 'roses', 'occasion', 'te', 'pleaded', 'shutoffs', 'surrounded', 'deserted', 'escalating', 'cd', 'stabilise', 'origin', 'nhsthankyou', 'oilsands', 'grappling', 'effecting', 'brothers', 'accommodations', 'ben', 'insolvency', 'hamburger', 'sacramento', 'disorder', 'metalminer', 'twat', 'sd', 'proxy', 'telcos', 'educated', 'master', 'easyuk', 'bollocks', 'greg', 'ipsos', 'herbs', 'restored', 'mayors', 'shopify', 'mongering', 'dontpanicbuy', 'toy', 'shelve', 'interrupted', 'covoid', 'blues', 'siblings', 'frank', 'fluctuate', 'multiply', 'deem', 'phases', 'shielded', 'surveillance', 'smashed', 'strips', 'melt', 'newday', 'nameandshame', 'lax', 'voluntarily', 'selves', 'yearly', 'escalated', 'hysterical', 'anthony', 'cartons', 'weary', 'cspi', 'troubling', 'deteriorating', 'mufc', 'edinburgh', 'quiroga', 'shaky', 'subscriber', 'downgrade', 'criticism', 'emma', 'overbuying', 'framework', 'coordinate', 'reversed', 'infuriating', 'studio', 'socialcare', 'freedoms', 'stockpiles', 'fury', 'pops', 'roy', 'gfc', 'swipe', 'za', 'unfold', 'concessional', 'harsh', 'viewers', 'essex', 'idiocy', 'rio', 'coronavirusitalianews', 'businessmen', 'embarrassing', 'jose', 'billgates', 'bang', 'scrub', 'pastor', 'spurs', 'established', 'removes', 'ninja', 'merchant', 'dashboard', 'pakistani', 'euets', 'repayment', 'consulting', 'supervalu', 'uninterrupted', 'freelancers', 'undoubtedly', 'transplant', 'liver', 'intel', 'changer', 'bookmark', 'whichuk', 'landed', 'tht', 'capes', 'musk', 'tackled', 'nails', 'dictate', 'heels', 'awaiting', 'condemn', 'spur', 'chemistry', 'tricky', 'ken', 'rebounds', 'muffins', 'metric', 'sw', 'withdraw', 'horrifying', 'mrs', 'remoteworking', 'cabinets', 'naija', 'stacked', 'bubbles', 'resumes', 'safehands', 'bargaining', 'refrigerator', 'unclear', 'tweaking', 'unveils', 'ding', 'interacting', 'realities', 'councils', 'shitting', 'upgrade', 'populated', 'spurred', 'ravages', 'cuban', 'explanation', 'revoke', 'establishment', 'nuclear', 'coma', 'grey', 'carer', 'imposing', 'overworked', 'carter', 'acquire', 'bruce', 'advantages', 'grounded', 'subsidies', 'qantas', 'statutory', 'sneak', 'thermal', 'anthonymace', 'oilpatch', 'homelessness', 'audiences', 'liberty', 'chronicles', 'heed', 'fry', 'punishing', 'harold', 'theellenshow', 'secured', 'aides', 'lancashire', 'catchup', 'criteria', 'germans', 'earners', 'demonstrating', 'lit', 'ms', 'blanket', 'jar', 'saves', 'chats', 'squirt', 'female', 'indonesian', 'cups', 'mill', 'everly', 'ties', 'revealing', 'abs', 'policemen', 'nigel', 'arses', 'resellers', 'votes', 'petrochemicals', 'punish', 'eager', 'cf', 'foreclosure', 'consultation', 'msg', 'presses', 'newsalert', 'iv', 'patrol', 'ems', 'ppeshortage', 'proudly', 'painkiller', 'duck', 'philadelphia', 'cnbctv', 'unsure', 'tb', 'underpaid', 'resolved', 'contacting', 'trace', 'roasted', 'captify', 'pros', 'positioned', 'awkward', 'occupied', 'eric', 'govwhitmer', 'conditioned', 'manages', 'rotting', 'persist', 'saturdays', 'drying', 'posters', 'hotspot', 'unpack', 'presentation', 'dose', 'upended', 'xauusd', 'keen', 'nursery', 'rewarded', 'enroll', 'prevail', 'jagograhakjago', 'queing', 'ruined', 'yoursafetyismysafety', 'cbcnews', 'pandemonium', 'businessowner', 'knocking', 'periods', 'cad', 'wand', 'jk', 'idiotic', 'boosted', 'scoop', 'patent', 'expressing', 'disadvantaged', 'blatant', 'reshape', 'mvp', 'madmax', 'ali', 'playstation', 'broccoli', 'boomer', 'attendant', 'ka', 'carlos', 'replies', 'toast', 'curry', 'weareinthistogether', 'consult', 'metpoliceuk', 'obamacare', 'professions', 'kwh', 'default', 'casual', 'blew', 'quarters', 'heartwarming', 'clash', 'tsunami', 'travels', 'mines', 'eradicate', 'documented', 'buckwheat', 'omfg', 'contaminating', 'intensify', 'morgue', 'bulkbuying', 'absent', 'govevers', 'accountable', 'vc', 'eco', 'maid', 'console', 'preying', 'protectthevulnerable', 'heaven', 'loonie', 'tweeps', 'reverse', 'shite', 'airfare', 'miguel', 'deposits', 'preserving', 'wil', 'myth', 'cast', 'triggering', 'generating', 'permit', 'oyo', 'precarious', 'govofco', 'independently', 'breast', 'bayarea', 'shorten', 'rumor', 'vertical', 'diversify', 'investigates', 'hudson', 'exhausting', 'vulnerabilities', 'geopolitical', 'diff', 'outreach', 'cdnecon', 'covididiot', 'teeth', 'cdt', 'pummeled', 'tipped', 'agbecerra', 'lockdownsa', 'mia', 'chad', 'northam', 'rife', 'totallockdown', 'align', 'ol', 'jharkhand', 'hog', 'sportsdirectuk', 'bahrain', 'correlation', 'forbearance', 'comparison', 'phosphate', 'capture', 'daca', 'boycottsportsdirect', 'narendra', 'nh', 'propping', 'covidactnow', 'postage', 'maxwell', 'hemantsorenjmm', 'liters', 'forest', 'cleanse', 'connecticut', 'samsung', 'jay', 'cereals', 'senschumer', 'fisheries', 'interstate', 'defence', 'chop', 'auchan', 'baltimore', 'veterans', 'pete', 'hv', 'separately', 'rallied', 'barr', 'blessings', 'prod', 'wider', 'surgeon', 'mechanisms', 'famine', 'yup', 'sterilizer', 'itc', 'samsclub', 'accessibility', 'holidaymakers', 'xbox', 'obsessed', 'bbq', 'belongs', 'flout', 'overcoming', 'weakening', 'surpass', 'vodafonegroup', 'transported', 'negotiating', 'lungs', 'adverse', 'gdcampaigns', 'apnea', 'parallel', 'protests', 'trumppressbriefing', 'elmhurst', 'bpd', 'consultancy', 'cartels', 'restart', 'moredays', 'pavement', 'losers', 'halifax', 'ahmedabad', 'happyeaster', 'reasi', 'unruly', 'waiver', 'suppresses', 'stagnant', 'markup', 'dha', 'rewrote', 'irs', 'boc', 'cong', 'allstate', 'sterile', 'exxon', 'strains', 'cuny', 'alleging', 'pi', 'cornell', 'draw', 'consistency', 'vaping', 'perceived', 'rotorua', 'napa', 'waitlist', 'saharan', 'delicate', 'aapl', 'staysafeug', 'activist', 'pedestrian', 'iot', 'trumppressconf', 'huntsman', 'dole', 'faulty', 'kpmg', 'explodes', 'feud', 'seanhannity', 'clapforthenhs', 'propylene', 'policing', 'kiwis', 'mrjamesob', 'filtration', 'withdraws', 'moderate', 'offerings', 'gambling', 'negotiated', 'eliminating', 'internationaldayofhappiness', 'festivals', 'foodie', 'booth', 'casually', 'whichever', 'rachelwharton', 'mcorkery', 'encouragement', 'santizer', 'invade', 'motivation', 'togetherwecan', 'riverside', 'shakes', 'behavioural', 'sliding', 'peddling', 'experian', 'paramedic', 'cheque', 'tri', 'beneath', 'romance', 'peaked', 'echo', 'bbcpolitics', 'bullet', 'unprepared', 'matched', 'propertymarket', 'tradingstandards', 'globalization', 'liked', 'invaluable', 'quaratineandchill', 'lawn', 'prohibited', 'newsroom', 'norway', 'normality', 'interior', 'sl', 'screwing', 'finest', 'thirds', 'asthmatic', 'shoulders', 'cornoravirusuk', 'xx', 'vox', 'compiling', 'jus', 'independence', 'ins', 'electricitymarkets', 'prone', 'coronaviruscrisis', 'busiest', 'obligation', 'continental', 'brighton', 'applauded', 'des', 'fortnite', 'chan', 'haram', 'imagination', 'contributed', 'representative', 'dietary', 'quadrupled', 'limbo', 'remarkable', 'comp', 'expendable', 'pushback', 'lengths', 'husbands', 'abhabhattarai', 'dent', 'taobao', 'mancity', 'shorter', 'mco', 'considerable', 'drains', 'rupees', 'appearance', 'generosity', 'mixing', 'ghanaians', 'agric', 'blossom', 'licks', 'drunk', 'comms', 'survivors', 'revoked', 'belgian', 'globalpandemic', 'capitalists', 'deflationary', 'mu', 'scarves', 'input', 'vinyl', 'generational', 'disneyland', 'spreaker', 'ge', 'houseprices', 'lockout', 'modelling', 'peaceful', 'upgrading', 'dean', 'spotify', 'academy', 'washhands', 'cabinfever', 'sth', 'throng', 'fairer', 'recycled', 'involve', 'operated', 'refinery', 'vilas', 'newyorkstateag', 'feds', 'mtnza', 'bbcworld', 'nicolasturgeon', 'boxed', 'cheering', 'flushable', 'officeofut', 'forecasting', 'modest', 'sits', 'teenage', 'bcs', 'dhscgovuk', 'capabilities', 'kenyatta', 'motorists', 'os', 'montreal', 'teaches', 'themed', 'overly', 'messing', 'inhumane', 'interrupt', 'exacerbate', 'psychological', 'trinidad', 'postponing', 'props', 'notify', 'penguin', 'adventures', 'superior', 'droplet', 'additionally', 'ep', 'acquiring', 'stretched', 'smo', 'yougov', 'tuesdays', 'bulletin', 'presidencyza', 'dod', 'nikki', 'terrorist', 'allocate', 'contrary', 'exemptions', 'nepal', 'effectiveness', 'essence', 'ssi', 'flagship', 'legends', 'yvr', 'democracy', 'peers', 'ra', 'sauces', 'emt', 'ecological', 'expiration', 'gig', 'supposedly', 'ubs', 'ham', 'eerie', 'attacked', 'honored', 'smokers', 'lightly', 'louder', 'monica', 'donuts', 'jo', 'dove', 'grandpa', 'wagers', 'degree', 'ski', 'quid', 'mintel', 'captain', 'conclusion', 'prepping', 'bug', 'sourced', 'oc', 'livestream', 'parked', 'shes', 'lengthy', 'favorites', 'anilsinghvi', 'glance', 'anticipating', 'toothpaste', 'regina', 'neworleans', 'penn', 'circular', 'centered', 'coronafighters', 'administrative', 'vons', 'ftse', 'traded', 'emergence', 'helpingothers', 'aussies', 'lunchtime', 'antiviral', 'migration', 'lasvegas', 'leo', 'unavoidable', 'sooo', 'happiness', 'laura', 'justify', 'airways', 'purse', 'reputation', 'stomach', 'walmartcanada', 'unusually', 'paradox', 'ineffective', 'diners', 'calming', 'ryanair', 'scaring', 'depleting', 'donaldjtrumpjr', 'santizers', 'businessnews', 'rwandatrade', 'albert', 'inspections', 'cspanwj', 'sore', 'projections', 'pritzker', 'supreme', 'voluntary', 'arsehole', 'resiliency', 'doordash', 'newspaper', 'preliminary', 'praises', 'patch', 'chefs', 'gf', 'scheduling', 'wga', 'lip', 'sterilize', 'tbh', 'uncomfortable', 'worrisome', 'labeled', 'inhaler', 'nephew', 'grind', 'nstnation', 'culinary', 'blast', 'purely', 'ness', 'resorted', 'smoothly', 'sausages', 'evans', 'dampen', 'digitaltransformation', 'shopee', 'barter', 'dickheads', 'credible', 'vlog', 'gregabbott', 'preview', 'maria', 'mishra', 'ralphs', 'chatter', 'cleansing', 'europeans', 'sbs', 'verified', 'counterfeit', 'tofu', 'voices', 'prize', 'fo', 'converting', 'maids', 'crushing', 'subway', 'platinum', 'proposing', 'demise', 'comforting', 'peterborough', 'frightened', 'overstock', 'congressional', 'empire', 'oversold', 'ivankatrump', 'laguna', 'southeast', 'ifpri', 'craig', 'identifying', 'pl', 'disagree', 'soviet', 'background', 'nyclockdown', 'thu', 'liar', 'hancock', 'nasdaq', 'reconsider', 'distilledspirit', 'lentils', 'grocerydelivery', 'relates', 'parker', 'frontlineheroes', 'mentioning', 'shelfs', 'deaf', 'londoners', 'transmit', 'casinos', 'vector', 'disparity', 'weaken', 'coin', 'holed', 'laborers', 'edit', 'entrepreneurs', 'unprotected', 'obscene', 'consumergoods', 'refugee', 'vunerable', 'yikes', 'hazardous', 'unreasonable', 'hk', 'hamilton', 'creditors', 'academic', 'bath', 'unseen', 'invested', 'malaysian', 'societal', 'navy', 'revive', 'informing', 'pizzas', 'patrick', 'renewed', 'catholic', 'monitored', 'oshawa', 'dated', 'haunt', 'fucker', 'wears', 'forefront', 'smallbiz', 'assumptions', 'districts', 'foreigners', 'guilt', 'tempting', 'breaker', 'makemytrip', 'defeated', 'owen', 'easiest', 'kungflu', 'genz', 'grofers', 'stayconnected', 'secs', 'foolish', 'trumpliespeopledie', 'parody', 'unimaginable', 'award', 'september', 'guides', 'canadalockdown', 'lean', 'conscience', 'maskshortage', 'supportlocalbusiness', 'wit', 'amsterdam', 'burgers', 'climbing', 'appealed', 'selfcare', 'retailgazette', 'silence', 'naivas', 'irl', 'hcw', 'hammering', 'si', 'outing', 'hse', 'clapforourcarers', 'ramps', 'trustworthy', 'cybercriminals', 'keto', 'snapchat', 'otc', 'importer', 'reassess', 'meditation', 'mosul', 'abrupt', 'quicktake', 'vanpoli', 'jewelosco', 'dancing', 'renewal', 'deter', 'scoopit', 'annually', 'bookstores', 'jolly', 'crossed', 'sprint', 'quaratine', 'weakens', 'cooperatives', 'yemen', 'conflict', 'integrated', 'stocker', 'horribly', 'picnic', 'unaware', 'housekeeping', 'rioting', 'beverly', 'carnage', 'description', 'sums', 'greenwich', 'overcrowded', 'inclusive', 'propublica', 'gravity', 'acquisition', 'onwards', 'stakes', 'levity', 'postmates', 'forming', 'epic', 'globeandmail', 'edt', 'heartbroken', 'robinsons', 'racial', 'lineups', 'sustaining', 'resin', 'fork', 'reviewing', 'livid', 'pinch', 'councillor', 'boutiques', 'theories', 'indiafightcorona', 'plug', 'dreading', 'documents', 'vaccination', 'dementia', 'dial', 'argued', 'dailymail', 'disconnected', 'racine', 'bbcwatchdog', 'distress', 'epidemics', 'jnj', 'neighbouring', 'macron', 'doomed', 'indicator', 'cylinders', 'klopapier', 'dominicraab', 'fe', 'stayinghome', 'nada', 'stabilizes', 'coat', 'protip', 'gran', 'guarantees', 'trades', 'dollargeneral', 'sidewalk', 'avengers', 'fifty', 'firstresponders', 'component', 'cookie', 'segments', 'catherine', 'itch', 'continually', 'boat', 'southampton', 'coronachainscare', 'inflatable', 'bodega', 'leasing', 'abdul', 'sometime', 'lifebuoy', 'jai', 'barrie', 'beresponsible', 'chilling', 'interviewing', 'destinations', 'malaysians', 'distraction', 'highstreet', 'jkenney', 'opting', 'introvert', 'instability', 'writingcommunity', 'saturdaymotivation', 'disasters', 'benice', 'jackpot', 'diverse', 'matatus', 'mountains', 'statistic', 'spokesman', 'buylocal', 'insa', 'tightly', 'nih', 'dads', 'smm', 'switches', 'thereby', 'offenders', 'distressed', 'damascus', 'jd', 'separated', 'preston', 'citizensadvice', 'websitedesign', 'embracing', 'homebuyers', 'rebecca', 'lakhs', 'accidentally', 'manutd', 'naps', 'lad', 'parasites', 'transferred', 'judged', 'flash', 'cycles', 'polls', 'piers', 'oap', 'joggers', 'maths', 'roast', 'bsybjp', 'enquiries', 'seasoning', 'illustrates', 'nightclub', 'louise', 'compelling', 'policymakers', 'costume', 'makinde', 'emergesmarter', 'mitigating', 'bo', 'volunteered', 'rebel', 'mgvcl', 'slight', 'cited', 'invading', 'remarks', 'flip', 'raymond', 'historically', 'corrected', 'stir', 'epicenter', 'picky', 'eater', 'labeling', 'harmed', 'scrambled', 'puzzles', 'healthtips', 'protesting', 'boycottvodafone', 'asleep', 'cult', 'norms', 'cerb', 'uncover', 'realty', 'stones', 'furloughing', 'instruct', 'qu', 'retailweek', 'spacex', 'poland', 'assaulted', 'denatured', 'beekeeping', 'advisers', 'falcone', 'sheriff', 'doorknobs', 'galway', 'greek', 'wings', 'consumerinsights', 'trumpliesamericansdie', 'ncdc', 'wheelchair', 'developers', 'ftcscambingo', 'thankyounhs', 'sykescottages', 'graphics', 'accusations', 'complexity', 'baseline', 'firmly', 'extensions', 'compounding', 'converts', 'remodels', 'cycleways', 'cautiously', 'eases', 'complained', 'accelerates', 'packers', 'reacted', 'stretching', 'contractors', 'pent', 'tiger', 'distributions', 'theater', 'txsu', 'lil', 'militias', 'milwaukee', 'engines', 'inconvenient', 'ppv', 'novartis', 'exxonmobil', 'jenkins', 'bridport', 'palmsunday', 'cougher', 'princess', 'deb', 'canibrands', 'wtop', 'catapulted', 'jasoosshow', 'easterbunny', 'kolonya', 'retaildive', 'espionage', 'affiliate', 'foodsystems', 'bedevils', 'gambia', 'jeremy', 'recalls', 'secrecy', 'mikequindazzi', 'vending', 'refuge', 'overreaction', 'ruby', 'specialty', 'fighters', 'pudding', 'recruited', 'superpower', 'scraps', 'crumbled', 'rapaport', 'defined', 'unknowingly', 'swept', 'lilly', 'foodprices', 'pmi', 'zoomupyourlife', 'upbeat', 'lumber', 'socioeconomic', 'endangered', 'beatcovid', 'flocking', 'lighter', 'soy', 'fitch', 'mis', 'bothered', 'muppets', 'rahulgandhi', 'bajaj', 'onlineclasses', 'bands', 'referring', 'minofhealthug', 'spared', 'interestingly', 'kmart', 'elizabeth', 'alter', 'naturalgas', 'swings', 'enfield', 'disappearing', 'bicycles', 'junkie', 'truckdrivers', 'californiacoronavirus', 'inspection', 'protectionism', 'trickle', 'destroys', 'understood', 'jimmyfallon', 'sticker', 'optical', 'npdgroup', 'lion', 'fart', 'divorce', 'troubles', 'mississauga', 'wey', 'wetherspoon', 'rogers', 'equipments', 'doj', 'tito', 'sara', 'continuously', 'bumper', 'lockdownnz', 'tpci', 'respective', 'drank', 'kazakhstan', 'rex', 'carol', 'mummy', 'voter', 'bundle', 'profiles', 'plumbing', 'rightfully', 'betty', 'coordinating', 'asparagus', 'tamil', 'spicy', 'pride', 'aceng', 'payroll', 'lavender', 'handshake', 'ibadan', 'pvt', 'imp', 'reuse', 'savlon', 'hs', 'croozefmnews', 'chronically', 'earliest', 'reacts', 'sin', 'condo', 'nanny', 'calculate', 'clientele', 'mirza', 'justin', 'witch', 'reprieve', 'papa', 'tryna', 'coronaaustralia', 'starmer', 'sup', 'troy', 'harrow', 'dwnews', 'statues', 'theyre', 'simultaneous', 'infra', 'monkeys', 'trumpplague', 'visible', 'indicated', 'attended', 'thecounter', 'proactively', 'agrisa', 'amenities', 'payouts', 'tee', 'cfa', 'sealed', 'lynn', 'didiza', 'draft', 'electrical', 'emotions', 'emirates', 'telecommunications', 'crown', 'sharpest', 'printer', 'boats', 'quiz', 'gartner', 'strategist', 'ironically', 'describe', 'slaps', 'bases', 'recreation', 'incentives', 'monopolies', 'thenewnormal', 'tobago', 'heal', 'desires', 'drtedros', 'guild', 'placement', 'gianteagle', 'souls', 'sticky', 'rosie', 'engine', 'caller', 'emotion', 'penne', 'deserving', 'predominantly', 'deforestation', 'dubbed', 'hampers', 'internationally', 'ont', 'washhand', 'tightening', 'enrollment', 'cologne', 'repairs', 'consumerbehaviour', 'retreat', 'pooping', 'outer', 'cody', 'warrenton', 'enduring', 'liquids', 'invention', 'flags', 'versova', 'certification', 'sofa', 'drawings', 'profs', 'establishes', 'tutorial', 'mitch', 'underwear', 'douglas', 'roommate', 'rum', 'consolidation', 'citrus', 'consumerism', 'competent', 'lehman', 'nonexistent', 'exploding', 'detected', 'symptomatic', 'ringing', 'scourge', 'character', 'shilla', 'lucrative', 'flushed', 'robbed', 'selfdistancing', 'proceed', 'locking', 'deodorant', 'bbcyourquestions', 'binmen', 'marginal', 'dense', 'von', 'campaigners', 'triage', 'freshdirect', 'hillaryclinton', 'dwp', 'waits', 'oldest', 'wardrobe', 'crackers', 'flattened', 'rank', 'southwestair', 'johncornyn', 'swamped', 'coronaviruske', 'spite', 'aca', 'lounge', 'bros', 'sophie', 'bearing', 'civic', 'manipulating', 'cpho', 'sustained', 'banging', 'tues', 'mandates', 'stanley', 'publish', 'bts', 'dishes', 'carona', 'waving', 'fuelled', 'itwire', 'indicators', 'carries', 'implies', 'healthcareworkers', 'expats', 'metlife', 'summit', 'unnoticed', 'pensioner', 'sorts', 'playgrounds', 'shook', 'katie', 'compassionate', 'scamming', 'principle', 'upsetting', 'survives', 'pertaining', 'unbelievably', 'sensanders', 'jon', 'crave', 'spacing', 'poster', 'fil', 'ego', 'subsidized', 'picks', 'universe', 'horrified', 'rmb', 'healing', 'jammu', 'hires', 'passwords', 'acct', 'nailed', 'dispose', 'denies', 'styles', 'unsold', 'newsaus', 'transforming', 'unleashed', 'charter', 'ir', 'twitch', 'departments', 'presidents', 'thismorning', 'contributes', 'pressing', 'intent', 'damages', 'canal', 'bev', 'drsanjaygupta', 'rocketed', 'tbt', 'defensive', 'praising', 'physician', 'lyon', 'embarrassment', 'quedateencasa', 'deceptive', 'reg', 'tolerate', 'trenches', 'height', 'mince', 'bathandbodyworks', 'corrections', 'institutional', 'steven', 'trumpcrash', 'acid', 'antibiotics', 'ic', 'saveworkers', 'dots', 'harassing', 'tranquileyes', 'covod', 'superstar', 'briefly', 'cons', 'tony', 'sept', 'bundles', 'jumping', 'toyota', 'timed', 'ravaged', 'libraries', 'wto', 'emerges', 'improvise', 'portland', 'interventions', 'leap', 'suppressed', 'explosive', 'dispenser', 'exists', 'paramount', 'wednesdaymotivation', 'subside', 'circles', 'ingrahamangle', 'wrecked', 'manual', 'leonard', 'native', 'impression', 'upped', 'kitco', 'hoboken', 'crappy', 'slumped', 'blogging', 'injection', 'angst', 'supportsmallbusiness', 'kings', 'bullion', 'bu', 'outrage', 'rupee', 'intake', 'sonny', 'diaries', 'maximize', 'ruling', 'ukrainian', 'foam', 'logistic', 'majorly', 'ransom', 'chilled', 'hmoindia', 'askdrh', 'continuous', 'sip', 'cub', 'crippling', 'penalty', 'custard', 'basmati', 'ideally', 'dips', 'lifts', 'planes', 'guideline', 'vintage', 'rtenews', 'infant', 'fighter', 'linings', 'pact', 'whomever', 'optimum', 'sleeves', 'govern', 'cease', 'wreaks', 'bailey', 'singer', 'tubs', 'capability', 'zeebusiness', 'coins', 'carryout', 'gavin', 'pressured', 'po', 'outfits', 'inexcusable', 'puerto', 'urine', 'educators', 'roulette', 'ga', 'lender', 'gem', 'vacancies', 'touting', 'bipartisan', 'kurdistan', 'clicking', 'devastation', 'grabs', 'battery', 'depriving', 'ghee', 'breach', 'existed', 'peel', 'external', 'chores', 'tele', 'widening', 'educating', 'hangs', 'togetherness', 'foil', 'randpaul', 'dynamics', 'script', 'hotlink', 'fccc', 'telkomza', 'clarification', 'uneasy', 'bury', 'examine', 'query', 'exponential', 'adversely', 'miserably', 'helpless', 'stubborn', 'squeezed', 'roaming', 'fasting', 'assuring', 'blueyonder', 'taxis', 'halts', 'fml', 'chrismurphyct', 'rogue', 'hella', 'mixture', 'fireworks', 'gro', 'taxation', 'cincinnati', 'presumably', 'nut', 'squad', 'stan', 'hills', 'diversification', 'perilofafrica', 'almond', 'concentration', 'seize', 'chocolates', 'anne', 'disrespectful', 'winco', 'bricks', 'vt', 'warranty', 'eventual', 'lg', 'intolerances', 'spreaders', 'erupted', 'siege', 'norwegian', 'coronakrise', 'hai', 'oranges', 'adams', 'takeover', 'implementation', 'inflationary', 'latimes', 'neat', 'radar', 'heath', 'sally', 'flag', 'overload', 'unitedkingdom', 'senategop', 'thankfulthursday', 'injustice', 'acccgovau', 'brookshire', 'precedent', 'busted', 'motor', 'coronavirustoronto', 'devastated', 'deterioration', 'dasani', 'sexy', 'hamptons', 'creams', 'matthewlesh', 'curtis', 'staysafeshoponline', 'civilised', 'midday', 'crafts', 'arts', 'coeliac', 'ritual', 'southkorea', 'mildly', 'edeka', 'invite', 'frauds', 'habra', 'epicentre', 'napkins', 'profited', 'bj', 'elusive', 'dock', 'costa', 'spinning', 'ds', 'confiscated', 'tgt', 'bouncers', 'dfs', 'borisout', 'desde', 'witham', 'scooters', 'sweets', 'dos', 'careless', 'throwbackthursday', 'buhari', 'mauritius', 'stayhomeaustralia', 'coronaviru', 'tasty', 'kano', 'escalation', 'brennan', 'refill', 'literacy', 'occidental', 'postman', 'indigenous', 'shattered', 'claire', 'dnc', 'growyourown', 'bcz', 'stopit', 'downgraded', 'allied', 'magecart', 'comical', 'booty', 'katrina', 'antimalarial', 'mygovindia', 'responsive', 'economical', 'pymnts', 'ethereum', 'sachs', 'niagara', 'median', 'thrilled', 'bothering', 'gown', 'canadacovid', 'puertorico', 'challenged', 'precisely', 'undermining', 'calockdown', 'hq', 'champions', 'nab', 'await', 'jefferson', 'cultural', 'hotlines', 'undeniable', 'rollercoaster', 'batman', 'boeing', 'existential', 'ttf', 'sewage', 'cram', 'prudent', 'shoved', 'liquefied', 'macro', 'wrestlemania', 'iranians', 'hongkong', 'ruled', 'hm', 'analyse', 'dread', 'bursting', 'hedging', 'catalyst', 'coach', 'cochrane', 'consultations', 'pac', 'invasion', 'dudes', 'counterfeiters', 'draining', 'appointed', 'queries', 'amwriting', 'repeats', 'sugary', 'creation', 'exempted', 'idaho', 'debut', 'diarrhoea', 'dstv', 'streams', 'zombieland', 'handbag', 'quilted', 'coronainmaharashtra', 'pickle', 'itv', 'drums', 'desi', 'highways', 'cellphone', 'representation', 'newsnight', 'juliahb', 'dreaming', 'newcastle', 'marty', 'morningsmaria', 'knight', 'namaste', 'handgel', 'gentleman', 'menace', 'ambassador', 'tabs', 'naughty', 'violent', 'arise', 'evaluation', 'publications', 'sha', 'irvine', 'clarke', 'slid', 'promotes', 'afar', 'roots', 'influenced', 'govpritzker', 'silicon', 'flourish', 'myers', 'applauds', 'rev', 'rains', 'confronting', 'aiding', 'fraser', 'boycotted', 'gasbuddy', 'pmmodi', 'appeals', 'comrade', 'consoles', 'coronavirusnyc', 'root', 'remembering', 'fraction', 'offence', 'bec', 'rylan', 'bullets', 'wawa', 'instances', 'crafting', 'duncan', 'rumour', 'efficiency', 'parenting', 'crisps', 'maskup', 'bush', 'furiously', 'colleges', 'reviewed', 'procedure', 'croydon', 'existent', 'adexchanger', 'lingering', 'prisoners', 'hefty', 'valuations', 'replenishing', 'rags', 'pat', 'bumped', 'detained', 'institution', 'blvd', 'keypads', 'lincoln', 'citi', 'pal', 'stockmarkets', 'janitorial', 'lovers', 'translate', 'oxford', 'bottoms', 'supermercados', 'lasted', 'ada', 'contra', 'visibly', 'designate', 'lame', 'copies', 'occasions', 'storefront', 'prediction', 'heri', 'flirting', 'handshakes', 'loblaw', 'messed', 'taps', 'agreements', 'admiration', 'fcacan', 'firstdayofspring', 'functions', 'collaborate', 'keepsafe', 'drilling', 'dries', 'tahini', 'commute', 'hindered', 'etsy', 'fab', 'slumps', 'scream', 'gi', 'lb', 'sufferers', 'loom', 'lobbies', 'indispensable', 'cauliflower', 'dhaka', 'cam', 'mastercard', 'museum', 'embarrassed', 'loudly', 'builders', 'zoonotic', 'wartime', 'dragging', 'dreaded', 'fade', 'backdrop', 'factsnotfear', 'hat', 'awake', 'pillows', 'selfishly', 'barcode', 'batteries', 'scroll', 'congregating']
Explanation#
Finding the embedding of a given word can be useful when we’re trying to represent sentences as a collection of word embeddings, like when we’re trying to make a weight matrix for the embedding layer of a network:
print( w2v.wv['computer'] )
[ 1.56678483e-01 -5.47299605e-05 -3.72928753e-02 5.50866686e-02
7.50368042e-03 -3.81420881e-01 -2.03134537e-01 1.82285398e-01
1.70147002e-01 3.55972201e-02 -9.29551497e-02 1.15267746e-01
9.77725442e-03 3.21065545e-01 3.13991427e-01 -1.12642623e-01
1.93580851e-01 -1.90923110e-01 2.17850208e-02 -4.48047698e-01
4.46203381e-01 7.81382173e-02 2.13342145e-01 -8.99965242e-02
-6.69273660e-02 -1.31833419e-01 -3.77602220e-01 -1.64268270e-01
-4.89765685e-03 -1.64141003e-02 2.36842021e-01 1.60418421e-01
-1.15571104e-01 -9.12075937e-02 7.50637054e-02 2.21435800e-01
-2.97262102e-01 -2.61366814e-01 -1.19328648e-01 -1.20876290e-01
-3.10746580e-01 -1.30184025e-01 -1.32084072e-01 -3.70754637e-02
1.73525080e-01 -4.12524827e-02 1.11855023e-01 6.77329376e-02
-3.57940197e-02 3.15948963e-01 2.59706289e-01 -9.83724520e-02
-4.93154898e-02 -1.12827934e-01 -1.03739135e-01 -8.83174464e-02
-1.44716734e-02 -1.91785954e-03 1.50307894e-01 7.53741935e-02
-8.44971016e-02 3.24151566e-04 2.92973015e-02 1.89461321e-01
-2.81258494e-01 1.51606798e-01 1.49220794e-01 2.57280409e-01
-9.04605836e-02 2.46678203e-01 1.23065142e-02 -6.96600825e-02
2.81806141e-01 -2.34010711e-01 6.52933195e-02 1.34839684e-01
-3.08356643e-01 2.82248072e-02 -3.78539115e-02 2.37508446e-01
2.73912288e-02 -3.22782546e-01 -2.85511225e-01 9.30902958e-02
2.82843173e-01 -4.35131751e-02 6.82438584e-03 2.83953488e-01
-8.77174512e-02 1.67258531e-01 8.78421888e-02 4.75272723e-02
1.39186144e-01 -1.14126846e-01 3.05977881e-01 6.92355409e-02
6.74140006e-02 1.04344040e-01 -3.65192980e-01 -1.29062086e-01]
Explanation#
We can also find out the similarity between given words (the cosine distance between their vectors):
w2v.wv.similarity('vladimir', 'putin')
0.82377356
w2v.wv.similarity('vladimir', 'modi')
0.67065156
Explanation#
With the gensim, we can also find the most similar words to a given word:
print(w2v.wv.most_similar('pay'))
[('paid', 0.6858607530593872), ('paying', 0.6821929812431335), ('wages', 0.6271983981132507), ('raise', 0.6147904396057129), ('bills', 0.6121124625205994), ('benefits', 0.581198513507843), ('receive', 0.5787360668182373), ('rent', 0.5779651999473572), ('hazard', 0.5580592155456543), ('wage', 0.5553852319717407)]
print(w2v.wv.most_similar('covid'))
[('coronavirus', 0.6121239066123962), ('virus', 0.5078110098838806), ('corona', 0.4850302040576935), ('coronaviruschallenge', 0.46205270290374756), ('coronacrisis', 0.4613567590713501), ('covidbc', 0.4549438953399658), ('convid', 0.45207855105400085), ('coronavirusindia', 0.4475623667240143), ('coronavirusoutbreakindia', 0.44667044281959534), ('coronaviruspandemic', 0.4427001476287842)]
print(w2v.wv.most_similar('india'))
[('nigeria', 0.7502180337905884), ('pakistan', 0.7422486543655396), ('narendramodi', 0.6548005938529968), ('drharshvardhan', 0.6406432390213013), ('amitshah', 0.6349469423294067), ('kenya', 0.6287263631820679), ('pmoindia', 0.628250777721405), ('ksa', 0.6237217783927917), ('irvpaswan', 0.6234133243560791), ('iran', 0.616048276424408)]
Explanation#
Similarly, we can use the same function to find analogies of the form: if x:y, then z:?. Here we enter the known relation x,y in the positive parameter, and the term who’s analogy has to be found in the negative parameter:
print(w2v.wv.most_similar(positive=['russian', 'russia'], negative=['arab']))
[('saudi', 0.7939720749855042), ('arabia', 0.7774107456207275), ('putin', 0.737236499786377), ('opec', 0.7016716599464417), ('trump', 0.6634055972099304), ('iran', 0.6270323395729065), ('agreed', 0.6244860887527466), ('oil', 0.620878279209137), ('mbs', 0.6121196150779724), ('saudis', 0.6068660616874695)]
Explanation#
We also have this method which works similar to an “odd one out” situation:
w2v.wv.doesnt_match(['grocery', 'covid', 'coronavirus'])
'grocery'
Visualising word vectors#
Word2Vec embeddings typically have dimensions of 100 or 300, making it impractical to visualize such high-dimensional spaces meaningfully. To address this, I used a code snippet from Stanford’s 224n course, which allows us to either input a list of words or specify the number of random samples to display. It then applies PCA to reduce the dimensionality, mapping the word vectors onto a 2D plane. The actual values on the axes aren’t important, as they don’t hold any specific meaning—what matters is that similar word vectors will appear closely clustered together.
from sklearn.decomposition import PCA
import matplotlib.pyplot as plt
import numpy as np
def display_pca_scatterplot(model, words=None, sample=0):
if words is None:
if sample > 0:
words = np.random.choice(list(model.wv.index_to_key), sample)
else:
words = model.wv.index_to_key
word_vectors = np.array([model.wv[w] for w in words])
twodim = PCA().fit_transform(word_vectors)[:,:2]
plt.figure(figsize=(6,6))
plt.scatter(twodim[:,0], twodim[:,1], edgecolors='k', c='r')
for word, (x,y) in zip(words, twodim):
plt.text(x+0.05, y+0.05, word)
display_pca_scatterplot(w2v, ['coronavirus', 'covid', 'virus', 'corona', 'disease', 'saudiarabia', 'doctor', 'hospital', 'pakistan', 'kenya',
'pay', 'paying', 'paid', 'wages', 'raise', 'bills', 'rent', 'charge'])
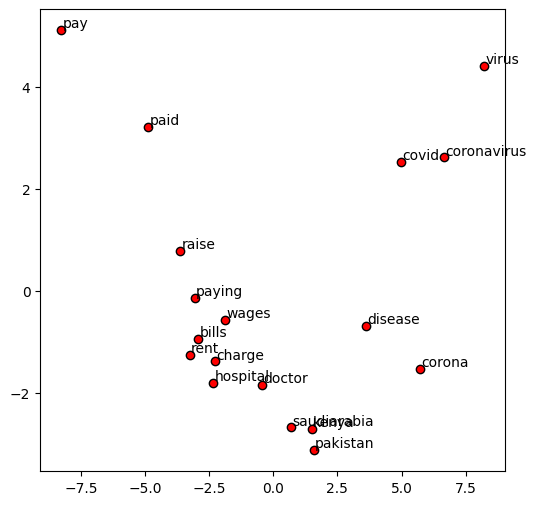
Why is Word2Vec revolutionary?#
Word2Vec’s powerful embeddings offer an advancement over earlier word vectorization methods like Latent Semantic Analysis (LSA), Singular Value Decomposition (SVD), and Global Vectors for Word Representation (GloVe), which existed before its introduction in 2013. Here’s why:
Word2Vec generates numerical vectors in a high-dimensional space while maintaining both semantic and syntactic relationships between words.
It outperforms its predecessors by simplifying the representation of relationships into fixed-sized vectors, reducing dimensionality, and making mathematical analysis more straightforward.
This technique is versatile, applicable to both textual and non-textual data, allowing for comparisons and similarity identification across a wide range of fields, from products and chemicals to genes and business concepts.
Word2Vec also supports training contextualized models using open-source code, optimized for speed and efficiency with specific datasets. For example, you can train a model on finance data if your target area is finance.
What are the limitations of Word2Vec?#
Has difficulty handling unknown words
One major limitation of Word2Vec is its inability to manage unknown or out-of-vocabulary words. If the model has not seen a word before, it cannot generate a proper vector, defaulting to a random one, which is suboptimal. This issue is especially pronounced in noisy data environments like Twitter, where certain words are used infrequently across a large dataset.
Doesn’t have shared representations at sub-word levels
Additionally, Word2Vec does not utilize shared representations at the sub-word level, treating each word as a standalone vector. This poses challenges for morphologically rich and polysynthetic languages like German, Turkish, and Arabic, where many words share similar morphological structures.
Difficult to scale to new languages
Scaling Word2Vec to new languages requires creating new embedding matrices. However, without the ability to share parameters, the model cannot be easily adapted for cross-lingual applications.
What are the applications of W2V?#
Word2Vec models are extensively utilized across various natural language processing applications. There are some of the most popular use cases.
Search Engines#
Word2Vec enhances the accuracy of search results. When a user poses a question, the search engine converts it into a vector representation. This vector is then compared to the representations of documents or web pages to find the most relevant matches. The algorithm also allows search engines to grasp the context of a query. For instance, if a user searches for “apple,” Word2Vec can determine whether they are interested in the fruit or the technology company based on the surrounding context.
Automated Translation#
Word2Vec is employed in automated translation systems, using graphical representations of word meanings across different languages. Well-known applications include Google Translate and Translate.com.
Customer Review Analysis#
Businesses can utilize Word2Vec to analyze thousands of customer reviews and extract valuable insights. By creating vector representations of words tailored to specific survey datasets, organizations can capture the intricate relationships between reviewed responses and their contexts. This data can be leveraged by machine learning algorithms for further analysis, enabling the identification of common themes and sentiments, which can inform strategies to address customer concerns.
Recommendation Systems#
Word2Vec is not limited to textual data; it can also be applied to any sequential data, such as user click sessions, search histories, and purchase records. This data can be harnessed to build effective recommender systems that enhance online business profitability by improving click-through rates and conversions. By generating fixed-sized vector representations for various items, such as places or products, the model captures human-like relationships and similarities between them. For example, by analyzing vector similarity scores among products, recommendations can be made for accessories or additional equipment, such as suggesting complementary sports gear to athletes purchasing a barbell. Airbnb, for instance, has successfully trained Word2Vec models on user click and conversion data to generate business value.